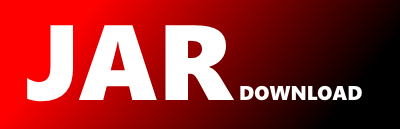
com.pulumi.googlenative.workloadmanager.v1.EvaluationArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.workloadmanager.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.workloadmanager.v1.inputs.ResourceFilterArgs;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EvaluationArgs extends com.pulumi.resources.ResourceArgs {
public static final EvaluationArgs Empty = new EvaluationArgs();
/**
* Description of the Evaluation
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return Description of the Evaluation
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy