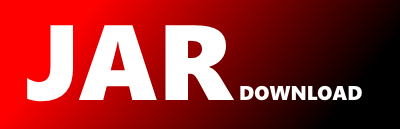
com.pulumi.googlenative.workstations.v1beta.inputs.GceInstanceArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.googlenative.workstations.v1beta.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.googlenative.workstations.v1beta.inputs.AcceleratorArgs;
import com.pulumi.googlenative.workstations.v1beta.inputs.GceConfidentialInstanceConfigArgs;
import com.pulumi.googlenative.workstations.v1beta.inputs.GceShieldedInstanceConfigArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A runtime using a Compute Engine instance.
*
*/
public final class GceInstanceArgs extends com.pulumi.resources.ResourceArgs {
public static final GceInstanceArgs Empty = new GceInstanceArgs();
/**
* A list of the type and count of accelerator cards attached to the instance.
*
*/
@Import(name="accelerators")
private @Nullable Output> accelerators;
/**
* @return A list of the type and count of accelerator cards attached to the instance.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy