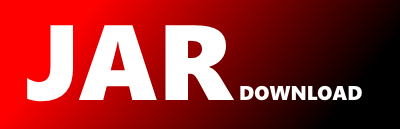
com.pulumi.ise.networkaccess.outputs.GetAuthorizationProfileResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ise Show documentation
Show all versions of ise Show documentation
A Pulumi package for managing resources on a Cisco ISE (Identity Service Engine) instance.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.ise.networkaccess.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.ise.networkaccess.outputs.GetAuthorizationProfileAdvancedAttribute;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetAuthorizationProfileResult {
/**
* @return Access type
*
*/
private String accessType;
/**
* @return ACL
*
*/
private String acl;
/**
* @return List of advanced attributes
*
*/
private List advancedAttributes;
/**
* @return Agentless Posture.
*
*/
private Boolean agentlessPosture;
/**
* @return Airespace ACL
*
*/
private String airespaceAcl;
/**
* @return Airespace IPv6 ACL
*
*/
private String airespaceIpv6Acl;
/**
* @return ASA VPN
*
*/
private String asaVpn;
/**
* @return Auto smart port
*
*/
private String autoSmartPort;
/**
* @return AVC profile
*
*/
private String avcProfile;
/**
* @return DACL name
*
*/
private String daclName;
/**
* @return Description
*
*/
private String description;
/**
* @return Easy wired session candidate
*
*/
private Boolean easywiredSessionCandidate;
/**
* @return The id of the object
*
*/
private String id;
/**
* @return Interface template
*
*/
private String interfaceTemplate;
/**
* @return IPv6 ACL
*
*/
private String ipv6AclFilter;
/**
* @return IPv6 DACL name
*
*/
private String ipv6DaclName;
/**
* @return MacSec policy
*
*/
private String macSecPolicy;
/**
* @return The name of the authorization profile
*
*/
private String name;
/**
* @return NEAT
*
*/
private Boolean neat;
/**
* @return Value needs to be an existing Network Device Profile
*
*/
private String profileName;
/**
* @return Maintain Connectivity During Reauthentication
*
*/
private String reauthenticationConnectivity;
/**
* @return Reauthentication timer
*
*/
private Integer reauthenticationTimer;
/**
* @return Service template
*
*/
private Boolean serviceTemplate;
/**
* @return Track movement
*
*/
private Boolean trackMovement;
/**
* @return Unique identifier
*
*/
private String uniqueIdentifier;
/**
* @return Vlan name or ID
*
*/
private String vlanNameId;
/**
* @return Vlan tag ID
*
*/
private Integer vlanTagId;
/**
* @return Voice domain permission
*
*/
private Boolean voiceDomainPermission;
/**
* @return Web authentication (local)
*
*/
private Boolean webAuth;
/**
* @return Web redirection ACL
*
*/
private String webRedirectionAcl;
/**
* @return This attribute is mandatory when `web_redirection_type` value is `CentralizedWebAuth`. For all other `web_redirection_type` values the field must be ignored.
*
*/
private Boolean webRedirectionDisplayCertificatesRenewalMessages;
/**
* @return A portal that exist in the DB and fits the `web_redirection_type`
*
*/
private String webRedirectionPortalName;
/**
* @return IP, hostname or FQDN
*
*/
private String webRedirectionStaticIpHostNameFqdn;
/**
* @return This type must fit the `web_redirection_portal_name`
*
*/
private String webRedirectionType;
private GetAuthorizationProfileResult() {}
/**
* @return Access type
*
*/
public String accessType() {
return this.accessType;
}
/**
* @return ACL
*
*/
public String acl() {
return this.acl;
}
/**
* @return List of advanced attributes
*
*/
public List advancedAttributes() {
return this.advancedAttributes;
}
/**
* @return Agentless Posture.
*
*/
public Boolean agentlessPosture() {
return this.agentlessPosture;
}
/**
* @return Airespace ACL
*
*/
public String airespaceAcl() {
return this.airespaceAcl;
}
/**
* @return Airespace IPv6 ACL
*
*/
public String airespaceIpv6Acl() {
return this.airespaceIpv6Acl;
}
/**
* @return ASA VPN
*
*/
public String asaVpn() {
return this.asaVpn;
}
/**
* @return Auto smart port
*
*/
public String autoSmartPort() {
return this.autoSmartPort;
}
/**
* @return AVC profile
*
*/
public String avcProfile() {
return this.avcProfile;
}
/**
* @return DACL name
*
*/
public String daclName() {
return this.daclName;
}
/**
* @return Description
*
*/
public String description() {
return this.description;
}
/**
* @return Easy wired session candidate
*
*/
public Boolean easywiredSessionCandidate() {
return this.easywiredSessionCandidate;
}
/**
* @return The id of the object
*
*/
public String id() {
return this.id;
}
/**
* @return Interface template
*
*/
public String interfaceTemplate() {
return this.interfaceTemplate;
}
/**
* @return IPv6 ACL
*
*/
public String ipv6AclFilter() {
return this.ipv6AclFilter;
}
/**
* @return IPv6 DACL name
*
*/
public String ipv6DaclName() {
return this.ipv6DaclName;
}
/**
* @return MacSec policy
*
*/
public String macSecPolicy() {
return this.macSecPolicy;
}
/**
* @return The name of the authorization profile
*
*/
public String name() {
return this.name;
}
/**
* @return NEAT
*
*/
public Boolean neat() {
return this.neat;
}
/**
* @return Value needs to be an existing Network Device Profile
*
*/
public String profileName() {
return this.profileName;
}
/**
* @return Maintain Connectivity During Reauthentication
*
*/
public String reauthenticationConnectivity() {
return this.reauthenticationConnectivity;
}
/**
* @return Reauthentication timer
*
*/
public Integer reauthenticationTimer() {
return this.reauthenticationTimer;
}
/**
* @return Service template
*
*/
public Boolean serviceTemplate() {
return this.serviceTemplate;
}
/**
* @return Track movement
*
*/
public Boolean trackMovement() {
return this.trackMovement;
}
/**
* @return Unique identifier
*
*/
public String uniqueIdentifier() {
return this.uniqueIdentifier;
}
/**
* @return Vlan name or ID
*
*/
public String vlanNameId() {
return this.vlanNameId;
}
/**
* @return Vlan tag ID
*
*/
public Integer vlanTagId() {
return this.vlanTagId;
}
/**
* @return Voice domain permission
*
*/
public Boolean voiceDomainPermission() {
return this.voiceDomainPermission;
}
/**
* @return Web authentication (local)
*
*/
public Boolean webAuth() {
return this.webAuth;
}
/**
* @return Web redirection ACL
*
*/
public String webRedirectionAcl() {
return this.webRedirectionAcl;
}
/**
* @return This attribute is mandatory when `web_redirection_type` value is `CentralizedWebAuth`. For all other `web_redirection_type` values the field must be ignored.
*
*/
public Boolean webRedirectionDisplayCertificatesRenewalMessages() {
return this.webRedirectionDisplayCertificatesRenewalMessages;
}
/**
* @return A portal that exist in the DB and fits the `web_redirection_type`
*
*/
public String webRedirectionPortalName() {
return this.webRedirectionPortalName;
}
/**
* @return IP, hostname or FQDN
*
*/
public String webRedirectionStaticIpHostNameFqdn() {
return this.webRedirectionStaticIpHostNameFqdn;
}
/**
* @return This type must fit the `web_redirection_portal_name`
*
*/
public String webRedirectionType() {
return this.webRedirectionType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAuthorizationProfileResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accessType;
private String acl;
private List advancedAttributes;
private Boolean agentlessPosture;
private String airespaceAcl;
private String airespaceIpv6Acl;
private String asaVpn;
private String autoSmartPort;
private String avcProfile;
private String daclName;
private String description;
private Boolean easywiredSessionCandidate;
private String id;
private String interfaceTemplate;
private String ipv6AclFilter;
private String ipv6DaclName;
private String macSecPolicy;
private String name;
private Boolean neat;
private String profileName;
private String reauthenticationConnectivity;
private Integer reauthenticationTimer;
private Boolean serviceTemplate;
private Boolean trackMovement;
private String uniqueIdentifier;
private String vlanNameId;
private Integer vlanTagId;
private Boolean voiceDomainPermission;
private Boolean webAuth;
private String webRedirectionAcl;
private Boolean webRedirectionDisplayCertificatesRenewalMessages;
private String webRedirectionPortalName;
private String webRedirectionStaticIpHostNameFqdn;
private String webRedirectionType;
public Builder() {}
public Builder(GetAuthorizationProfileResult defaults) {
Objects.requireNonNull(defaults);
this.accessType = defaults.accessType;
this.acl = defaults.acl;
this.advancedAttributes = defaults.advancedAttributes;
this.agentlessPosture = defaults.agentlessPosture;
this.airespaceAcl = defaults.airespaceAcl;
this.airespaceIpv6Acl = defaults.airespaceIpv6Acl;
this.asaVpn = defaults.asaVpn;
this.autoSmartPort = defaults.autoSmartPort;
this.avcProfile = defaults.avcProfile;
this.daclName = defaults.daclName;
this.description = defaults.description;
this.easywiredSessionCandidate = defaults.easywiredSessionCandidate;
this.id = defaults.id;
this.interfaceTemplate = defaults.interfaceTemplate;
this.ipv6AclFilter = defaults.ipv6AclFilter;
this.ipv6DaclName = defaults.ipv6DaclName;
this.macSecPolicy = defaults.macSecPolicy;
this.name = defaults.name;
this.neat = defaults.neat;
this.profileName = defaults.profileName;
this.reauthenticationConnectivity = defaults.reauthenticationConnectivity;
this.reauthenticationTimer = defaults.reauthenticationTimer;
this.serviceTemplate = defaults.serviceTemplate;
this.trackMovement = defaults.trackMovement;
this.uniqueIdentifier = defaults.uniqueIdentifier;
this.vlanNameId = defaults.vlanNameId;
this.vlanTagId = defaults.vlanTagId;
this.voiceDomainPermission = defaults.voiceDomainPermission;
this.webAuth = defaults.webAuth;
this.webRedirectionAcl = defaults.webRedirectionAcl;
this.webRedirectionDisplayCertificatesRenewalMessages = defaults.webRedirectionDisplayCertificatesRenewalMessages;
this.webRedirectionPortalName = defaults.webRedirectionPortalName;
this.webRedirectionStaticIpHostNameFqdn = defaults.webRedirectionStaticIpHostNameFqdn;
this.webRedirectionType = defaults.webRedirectionType;
}
@CustomType.Setter
public Builder accessType(String accessType) {
if (accessType == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "accessType");
}
this.accessType = accessType;
return this;
}
@CustomType.Setter
public Builder acl(String acl) {
if (acl == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "acl");
}
this.acl = acl;
return this;
}
@CustomType.Setter
public Builder advancedAttributes(List advancedAttributes) {
if (advancedAttributes == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "advancedAttributes");
}
this.advancedAttributes = advancedAttributes;
return this;
}
public Builder advancedAttributes(GetAuthorizationProfileAdvancedAttribute... advancedAttributes) {
return advancedAttributes(List.of(advancedAttributes));
}
@CustomType.Setter
public Builder agentlessPosture(Boolean agentlessPosture) {
if (agentlessPosture == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "agentlessPosture");
}
this.agentlessPosture = agentlessPosture;
return this;
}
@CustomType.Setter
public Builder airespaceAcl(String airespaceAcl) {
if (airespaceAcl == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "airespaceAcl");
}
this.airespaceAcl = airespaceAcl;
return this;
}
@CustomType.Setter
public Builder airespaceIpv6Acl(String airespaceIpv6Acl) {
if (airespaceIpv6Acl == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "airespaceIpv6Acl");
}
this.airespaceIpv6Acl = airespaceIpv6Acl;
return this;
}
@CustomType.Setter
public Builder asaVpn(String asaVpn) {
if (asaVpn == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "asaVpn");
}
this.asaVpn = asaVpn;
return this;
}
@CustomType.Setter
public Builder autoSmartPort(String autoSmartPort) {
if (autoSmartPort == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "autoSmartPort");
}
this.autoSmartPort = autoSmartPort;
return this;
}
@CustomType.Setter
public Builder avcProfile(String avcProfile) {
if (avcProfile == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "avcProfile");
}
this.avcProfile = avcProfile;
return this;
}
@CustomType.Setter
public Builder daclName(String daclName) {
if (daclName == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "daclName");
}
this.daclName = daclName;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder easywiredSessionCandidate(Boolean easywiredSessionCandidate) {
if (easywiredSessionCandidate == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "easywiredSessionCandidate");
}
this.easywiredSessionCandidate = easywiredSessionCandidate;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder interfaceTemplate(String interfaceTemplate) {
if (interfaceTemplate == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "interfaceTemplate");
}
this.interfaceTemplate = interfaceTemplate;
return this;
}
@CustomType.Setter
public Builder ipv6AclFilter(String ipv6AclFilter) {
if (ipv6AclFilter == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "ipv6AclFilter");
}
this.ipv6AclFilter = ipv6AclFilter;
return this;
}
@CustomType.Setter
public Builder ipv6DaclName(String ipv6DaclName) {
if (ipv6DaclName == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "ipv6DaclName");
}
this.ipv6DaclName = ipv6DaclName;
return this;
}
@CustomType.Setter
public Builder macSecPolicy(String macSecPolicy) {
if (macSecPolicy == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "macSecPolicy");
}
this.macSecPolicy = macSecPolicy;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder neat(Boolean neat) {
if (neat == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "neat");
}
this.neat = neat;
return this;
}
@CustomType.Setter
public Builder profileName(String profileName) {
if (profileName == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "profileName");
}
this.profileName = profileName;
return this;
}
@CustomType.Setter
public Builder reauthenticationConnectivity(String reauthenticationConnectivity) {
if (reauthenticationConnectivity == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "reauthenticationConnectivity");
}
this.reauthenticationConnectivity = reauthenticationConnectivity;
return this;
}
@CustomType.Setter
public Builder reauthenticationTimer(Integer reauthenticationTimer) {
if (reauthenticationTimer == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "reauthenticationTimer");
}
this.reauthenticationTimer = reauthenticationTimer;
return this;
}
@CustomType.Setter
public Builder serviceTemplate(Boolean serviceTemplate) {
if (serviceTemplate == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "serviceTemplate");
}
this.serviceTemplate = serviceTemplate;
return this;
}
@CustomType.Setter
public Builder trackMovement(Boolean trackMovement) {
if (trackMovement == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "trackMovement");
}
this.trackMovement = trackMovement;
return this;
}
@CustomType.Setter
public Builder uniqueIdentifier(String uniqueIdentifier) {
if (uniqueIdentifier == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "uniqueIdentifier");
}
this.uniqueIdentifier = uniqueIdentifier;
return this;
}
@CustomType.Setter
public Builder vlanNameId(String vlanNameId) {
if (vlanNameId == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "vlanNameId");
}
this.vlanNameId = vlanNameId;
return this;
}
@CustomType.Setter
public Builder vlanTagId(Integer vlanTagId) {
if (vlanTagId == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "vlanTagId");
}
this.vlanTagId = vlanTagId;
return this;
}
@CustomType.Setter
public Builder voiceDomainPermission(Boolean voiceDomainPermission) {
if (voiceDomainPermission == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "voiceDomainPermission");
}
this.voiceDomainPermission = voiceDomainPermission;
return this;
}
@CustomType.Setter
public Builder webAuth(Boolean webAuth) {
if (webAuth == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "webAuth");
}
this.webAuth = webAuth;
return this;
}
@CustomType.Setter
public Builder webRedirectionAcl(String webRedirectionAcl) {
if (webRedirectionAcl == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "webRedirectionAcl");
}
this.webRedirectionAcl = webRedirectionAcl;
return this;
}
@CustomType.Setter
public Builder webRedirectionDisplayCertificatesRenewalMessages(Boolean webRedirectionDisplayCertificatesRenewalMessages) {
if (webRedirectionDisplayCertificatesRenewalMessages == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "webRedirectionDisplayCertificatesRenewalMessages");
}
this.webRedirectionDisplayCertificatesRenewalMessages = webRedirectionDisplayCertificatesRenewalMessages;
return this;
}
@CustomType.Setter
public Builder webRedirectionPortalName(String webRedirectionPortalName) {
if (webRedirectionPortalName == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "webRedirectionPortalName");
}
this.webRedirectionPortalName = webRedirectionPortalName;
return this;
}
@CustomType.Setter
public Builder webRedirectionStaticIpHostNameFqdn(String webRedirectionStaticIpHostNameFqdn) {
if (webRedirectionStaticIpHostNameFqdn == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "webRedirectionStaticIpHostNameFqdn");
}
this.webRedirectionStaticIpHostNameFqdn = webRedirectionStaticIpHostNameFqdn;
return this;
}
@CustomType.Setter
public Builder webRedirectionType(String webRedirectionType) {
if (webRedirectionType == null) {
throw new MissingRequiredPropertyException("GetAuthorizationProfileResult", "webRedirectionType");
}
this.webRedirectionType = webRedirectionType;
return this;
}
public GetAuthorizationProfileResult build() {
final var _resultValue = new GetAuthorizationProfileResult();
_resultValue.accessType = accessType;
_resultValue.acl = acl;
_resultValue.advancedAttributes = advancedAttributes;
_resultValue.agentlessPosture = agentlessPosture;
_resultValue.airespaceAcl = airespaceAcl;
_resultValue.airespaceIpv6Acl = airespaceIpv6Acl;
_resultValue.asaVpn = asaVpn;
_resultValue.autoSmartPort = autoSmartPort;
_resultValue.avcProfile = avcProfile;
_resultValue.daclName = daclName;
_resultValue.description = description;
_resultValue.easywiredSessionCandidate = easywiredSessionCandidate;
_resultValue.id = id;
_resultValue.interfaceTemplate = interfaceTemplate;
_resultValue.ipv6AclFilter = ipv6AclFilter;
_resultValue.ipv6DaclName = ipv6DaclName;
_resultValue.macSecPolicy = macSecPolicy;
_resultValue.name = name;
_resultValue.neat = neat;
_resultValue.profileName = profileName;
_resultValue.reauthenticationConnectivity = reauthenticationConnectivity;
_resultValue.reauthenticationTimer = reauthenticationTimer;
_resultValue.serviceTemplate = serviceTemplate;
_resultValue.trackMovement = trackMovement;
_resultValue.uniqueIdentifier = uniqueIdentifier;
_resultValue.vlanNameId = vlanNameId;
_resultValue.vlanTagId = vlanTagId;
_resultValue.voiceDomainPermission = voiceDomainPermission;
_resultValue.webAuth = webAuth;
_resultValue.webRedirectionAcl = webRedirectionAcl;
_resultValue.webRedirectionDisplayCertificatesRenewalMessages = webRedirectionDisplayCertificatesRenewalMessages;
_resultValue.webRedirectionPortalName = webRedirectionPortalName;
_resultValue.webRedirectionStaticIpHostNameFqdn = webRedirectionStaticIpHostNameFqdn;
_resultValue.webRedirectionType = webRedirectionType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy