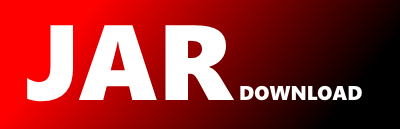
com.pulumi.ise.networkaccess.outputs.GetPolicySetResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ise Show documentation
Show all versions of ise Show documentation
A Pulumi package for managing resources on a Cisco ISE (Identity Service Engine) instance.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.ise.networkaccess.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.ise.networkaccess.outputs.GetPolicySetChildren;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetPolicySetResult {
/**
* @return List of child conditions. `condition_type` must be one of `ConditionAndBlock` or `ConditionOrBlock`.
*
*/
private List childrens;
/**
* @return Dictionary attribute name
*
*/
private String conditionAttributeName;
/**
* @return Attribute value for condition. Value type is specified in dictionary object.
*
*/
private String conditionAttributeValue;
/**
* @return Dictionary name
*
*/
private String conditionDictionaryName;
/**
* @return Dictionary value
*
*/
private String conditionDictionaryValue;
/**
* @return UUID for condition
*
*/
private String conditionId;
/**
* @return Indicates whereas this condition is in negate mode
*
*/
private Boolean conditionIsNegate;
/**
* @return Equality operator
*
*/
private String conditionOperator;
/**
* @return Indicates whether the record is the condition itself or a logical aggregation. Logical aggreation indicates that additional conditions are present under the children attribute.
*
*/
private String conditionType;
/**
* @return Indicates if this policy set is the default one
*
*/
private Boolean default_;
/**
* @return The description of the policy set
*
*/
private String description;
/**
* @return The id of the object
*
*/
private String id;
/**
* @return Flag which indicates if the policy set service is of type 'Proxy Sequence' or 'Allowed Protocols'
*
*/
private Boolean isProxy;
/**
* @return Given name for the policy set, [Valid characters are alphanumerics, underscore, hyphen, space, period, parentheses]
*
*/
private String name;
/**
* @return The rank (priority) in relation to other policy sets. Lower rank is higher priority.
*
*/
private Integer rank;
/**
* @return Policy set service identifier. 'Allowed Protocols' or 'Server Sequence'.
*
*/
private String serviceName;
/**
* @return The state that the policy set is in. A disabled policy set cannot be matched.
*
*/
private String state;
private GetPolicySetResult() {}
/**
* @return List of child conditions. `condition_type` must be one of `ConditionAndBlock` or `ConditionOrBlock`.
*
*/
public List childrens() {
return this.childrens;
}
/**
* @return Dictionary attribute name
*
*/
public String conditionAttributeName() {
return this.conditionAttributeName;
}
/**
* @return Attribute value for condition. Value type is specified in dictionary object.
*
*/
public String conditionAttributeValue() {
return this.conditionAttributeValue;
}
/**
* @return Dictionary name
*
*/
public String conditionDictionaryName() {
return this.conditionDictionaryName;
}
/**
* @return Dictionary value
*
*/
public String conditionDictionaryValue() {
return this.conditionDictionaryValue;
}
/**
* @return UUID for condition
*
*/
public String conditionId() {
return this.conditionId;
}
/**
* @return Indicates whereas this condition is in negate mode
*
*/
public Boolean conditionIsNegate() {
return this.conditionIsNegate;
}
/**
* @return Equality operator
*
*/
public String conditionOperator() {
return this.conditionOperator;
}
/**
* @return Indicates whether the record is the condition itself or a logical aggregation. Logical aggreation indicates that additional conditions are present under the children attribute.
*
*/
public String conditionType() {
return this.conditionType;
}
/**
* @return Indicates if this policy set is the default one
*
*/
public Boolean default_() {
return this.default_;
}
/**
* @return The description of the policy set
*
*/
public String description() {
return this.description;
}
/**
* @return The id of the object
*
*/
public String id() {
return this.id;
}
/**
* @return Flag which indicates if the policy set service is of type 'Proxy Sequence' or 'Allowed Protocols'
*
*/
public Boolean isProxy() {
return this.isProxy;
}
/**
* @return Given name for the policy set, [Valid characters are alphanumerics, underscore, hyphen, space, period, parentheses]
*
*/
public String name() {
return this.name;
}
/**
* @return The rank (priority) in relation to other policy sets. Lower rank is higher priority.
*
*/
public Integer rank() {
return this.rank;
}
/**
* @return Policy set service identifier. 'Allowed Protocols' or 'Server Sequence'.
*
*/
public String serviceName() {
return this.serviceName;
}
/**
* @return The state that the policy set is in. A disabled policy set cannot be matched.
*
*/
public String state() {
return this.state;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPolicySetResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List childrens;
private String conditionAttributeName;
private String conditionAttributeValue;
private String conditionDictionaryName;
private String conditionDictionaryValue;
private String conditionId;
private Boolean conditionIsNegate;
private String conditionOperator;
private String conditionType;
private Boolean default_;
private String description;
private String id;
private Boolean isProxy;
private String name;
private Integer rank;
private String serviceName;
private String state;
public Builder() {}
public Builder(GetPolicySetResult defaults) {
Objects.requireNonNull(defaults);
this.childrens = defaults.childrens;
this.conditionAttributeName = defaults.conditionAttributeName;
this.conditionAttributeValue = defaults.conditionAttributeValue;
this.conditionDictionaryName = defaults.conditionDictionaryName;
this.conditionDictionaryValue = defaults.conditionDictionaryValue;
this.conditionId = defaults.conditionId;
this.conditionIsNegate = defaults.conditionIsNegate;
this.conditionOperator = defaults.conditionOperator;
this.conditionType = defaults.conditionType;
this.default_ = defaults.default_;
this.description = defaults.description;
this.id = defaults.id;
this.isProxy = defaults.isProxy;
this.name = defaults.name;
this.rank = defaults.rank;
this.serviceName = defaults.serviceName;
this.state = defaults.state;
}
@CustomType.Setter
public Builder childrens(List childrens) {
if (childrens == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "childrens");
}
this.childrens = childrens;
return this;
}
public Builder childrens(GetPolicySetChildren... childrens) {
return childrens(List.of(childrens));
}
@CustomType.Setter
public Builder conditionAttributeName(String conditionAttributeName) {
if (conditionAttributeName == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "conditionAttributeName");
}
this.conditionAttributeName = conditionAttributeName;
return this;
}
@CustomType.Setter
public Builder conditionAttributeValue(String conditionAttributeValue) {
if (conditionAttributeValue == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "conditionAttributeValue");
}
this.conditionAttributeValue = conditionAttributeValue;
return this;
}
@CustomType.Setter
public Builder conditionDictionaryName(String conditionDictionaryName) {
if (conditionDictionaryName == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "conditionDictionaryName");
}
this.conditionDictionaryName = conditionDictionaryName;
return this;
}
@CustomType.Setter
public Builder conditionDictionaryValue(String conditionDictionaryValue) {
if (conditionDictionaryValue == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "conditionDictionaryValue");
}
this.conditionDictionaryValue = conditionDictionaryValue;
return this;
}
@CustomType.Setter
public Builder conditionId(String conditionId) {
if (conditionId == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "conditionId");
}
this.conditionId = conditionId;
return this;
}
@CustomType.Setter
public Builder conditionIsNegate(Boolean conditionIsNegate) {
if (conditionIsNegate == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "conditionIsNegate");
}
this.conditionIsNegate = conditionIsNegate;
return this;
}
@CustomType.Setter
public Builder conditionOperator(String conditionOperator) {
if (conditionOperator == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "conditionOperator");
}
this.conditionOperator = conditionOperator;
return this;
}
@CustomType.Setter
public Builder conditionType(String conditionType) {
if (conditionType == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "conditionType");
}
this.conditionType = conditionType;
return this;
}
@CustomType.Setter("default")
public Builder default_(Boolean default_) {
if (default_ == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "default_");
}
this.default_ = default_;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isProxy(Boolean isProxy) {
if (isProxy == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "isProxy");
}
this.isProxy = isProxy;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder rank(Integer rank) {
if (rank == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "rank");
}
this.rank = rank;
return this;
}
@CustomType.Setter
public Builder serviceName(String serviceName) {
if (serviceName == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "serviceName");
}
this.serviceName = serviceName;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetPolicySetResult", "state");
}
this.state = state;
return this;
}
public GetPolicySetResult build() {
final var _resultValue = new GetPolicySetResult();
_resultValue.childrens = childrens;
_resultValue.conditionAttributeName = conditionAttributeName;
_resultValue.conditionAttributeValue = conditionAttributeValue;
_resultValue.conditionDictionaryName = conditionDictionaryName;
_resultValue.conditionDictionaryValue = conditionDictionaryValue;
_resultValue.conditionId = conditionId;
_resultValue.conditionIsNegate = conditionIsNegate;
_resultValue.conditionOperator = conditionOperator;
_resultValue.conditionType = conditionType;
_resultValue.default_ = default_;
_resultValue.description = description;
_resultValue.id = id;
_resultValue.isProxy = isProxy;
_resultValue.name = name;
_resultValue.rank = rank;
_resultValue.serviceName = serviceName;
_resultValue.state = state;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy