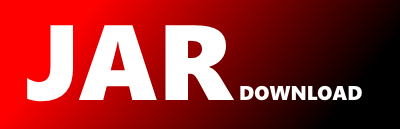
com.pulumi.junipermist.org.inputs.DeviceprofileGatewayBgpConfigArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.junipermist.org.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.junipermist.org.inputs.DeviceprofileGatewayBgpConfigCommunityArgs;
import com.pulumi.junipermist.org.inputs.DeviceprofileGatewayBgpConfigNeighborsArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DeviceprofileGatewayBgpConfigArgs extends com.pulumi.resources.ResourceArgs {
public static final DeviceprofileGatewayBgpConfigArgs Empty = new DeviceprofileGatewayBgpConfigArgs();
@Import(name="authKey")
private @Nullable Output authKey;
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy