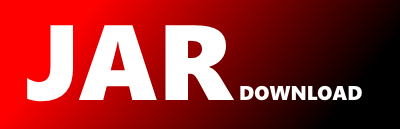
com.pulumi.junipermist.site.Webhook Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.junipermist.site;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.junipermist.Utilities;
import com.pulumi.junipermist.site.WebhookArgs;
import com.pulumi.junipermist.site.inputs.WebhookState;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* This resource manages Site Webhooks.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.junipermist.site.Webhook;
* import com.pulumi.junipermist.site.WebhookArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var webhookOne = new Webhook("webhookOne", WebhookArgs.builder()
* .siteId(terraformSite.id())
* .name("webhook_one")
* .type("http-post")
* .url("https://myserver.com:4321/")
* .verifyCert(false)
* .enabled(true)
* .topics(
* "device-events",
* "alarms",
* "audits",
* "client-join",
* "client-info",
* "client-sessions",
* "device-updowns",
* "mxedge-events",
* "nac-events",
* "nac-accounting")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `mist_site_webhook` with:
*
* Site Webhook can be imported by specifying the site_id and the webhook_id
*
* ```sh
* $ pulumi import junipermist:site/webhook:Webhook webhook_one 17b46405-3a6d-4715-8bb4-6bb6d06f316a.d3c42998-9012-4859-9743-6b9bee475309
* ```
*
*/
@ResourceType(type="junipermist:site/webhook:Webhook")
public class Webhook extends com.pulumi.resources.CustomResource {
/**
* whether webhook is enabled
*
*/
@Export(name="enabled", refs={Boolean.class}, tree="[0]")
private Output enabled;
/**
* @return whether webhook is enabled
*
*/
public Output enabled() {
return this.enabled;
}
/**
* if `type`=`http-post`, additional custom HTTP headers to add
* the headers name and value must be string, total bytes of headers name and value must be less than 1000
*
*/
@Export(name="headers", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> headers;
/**
* @return if `type`=`http-post`, additional custom HTTP headers to add
* the headers name and value must be string, total bytes of headers name and value must be less than 1000
*
*/
public Output>> headers() {
return Codegen.optional(this.headers);
}
/**
* name of the webhook
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return name of the webhook
*
*/
public Output name() {
return this.name;
}
/**
* required when `oauth2_grant_type`==`client_credentials`
*
*/
@Export(name="oauth2ClientId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> oauth2ClientId;
/**
* @return required when `oauth2_grant_type`==`client_credentials`
*
*/
public Output> oauth2ClientId() {
return Codegen.optional(this.oauth2ClientId);
}
/**
* required when `oauth2_grant_type`==`client_credentials`
*
*/
@Export(name="oauth2ClientSecret", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> oauth2ClientSecret;
/**
* @return required when `oauth2_grant_type`==`client_credentials`
*
*/
public Output> oauth2ClientSecret() {
return Codegen.optional(this.oauth2ClientSecret);
}
/**
* required when `type`==`oauth2`. enum: `client_credentials`, `password`
*
*/
@Export(name="oauth2GrantType", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> oauth2GrantType;
/**
* @return required when `type`==`oauth2`. enum: `client_credentials`, `password`
*
*/
public Output> oauth2GrantType() {
return Codegen.optional(this.oauth2GrantType);
}
/**
* required when `oauth2_grant_type`==`password`
*
*/
@Export(name="oauth2Password", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> oauth2Password;
/**
* @return required when `oauth2_grant_type`==`password`
*
*/
public Output> oauth2Password() {
return Codegen.optional(this.oauth2Password);
}
/**
* required when `type`==`oauth2`, if provided, will be used in the token request
*
*/
@Export(name="oauth2Scopes", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> oauth2Scopes;
/**
* @return required when `type`==`oauth2`, if provided, will be used in the token request
*
*/
public Output>> oauth2Scopes() {
return Codegen.optional(this.oauth2Scopes);
}
/**
* required when `type`==`oauth2`
*
*/
@Export(name="oauth2TokenUrl", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> oauth2TokenUrl;
/**
* @return required when `type`==`oauth2`
*
*/
public Output> oauth2TokenUrl() {
return Codegen.optional(this.oauth2TokenUrl);
}
/**
* required when `oauth2_grant_type`==`password`
*
*/
@Export(name="oauth2Username", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> oauth2Username;
/**
* @return required when `oauth2_grant_type`==`password`
*
*/
public Output> oauth2Username() {
return Codegen.optional(this.oauth2Username);
}
@Export(name="orgId", refs={String.class}, tree="[0]")
private Output orgId;
public Output orgId() {
return this.orgId;
}
/**
* only if `type`=`http-post`
*
*/
@Export(name="secret", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> secret;
/**
* @return only if `type`=`http-post`
*
*/
public Output> secret() {
return Codegen.optional(this.secret);
}
@Export(name="siteId", refs={String.class}, tree="[0]")
private Output siteId;
public Output siteId() {
return this.siteId;
}
/**
* required if `type`=`splunk` If splunk_token is not defined for a type Splunk webhook, it will not send, regardless if
* the webhook receiver is configured to accept it.'
*
*/
@Export(name="splunkToken", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> splunkToken;
/**
* @return required if `type`=`splunk` If splunk_token is not defined for a type Splunk webhook, it will not send, regardless if
* the webhook receiver is configured to accept it.'
*
*/
public Output> splunkToken() {
return Codegen.optional(this.splunkToken);
}
/**
* enum: `alarms`, `asset-raw`, `asset-raw-rssi`, `audits`, `client-info`, `client-join`, `client-latency`, `client-sessions`, `device-updowns`, `device-events`, `discovered-raw-rssi`, `location`, `location_asset`, `location_centrak`, `location_client`, `location_sdk`, `location_unclient`, `mxedge-events`, `nac-accounting`, `nac_events`, `occupancy-alerts`, `rssizone`, `sdkclient_scan_data`, `site_sle`, `vbeacon`, `wifi-conn-raw`, `wifi-unconn-raw`, `zone`
*
*/
@Export(name="topics", refs={List.class,String.class}, tree="[0,1]")
private Output> topics;
/**
* @return enum: `alarms`, `asset-raw`, `asset-raw-rssi`, `audits`, `client-info`, `client-join`, `client-latency`, `client-sessions`, `device-updowns`, `device-events`, `discovered-raw-rssi`, `location`, `location_asset`, `location_centrak`, `location_client`, `location_sdk`, `location_unclient`, `mxedge-events`, `nac-accounting`, `nac_events`, `occupancy-alerts`, `rssizone`, `sdkclient_scan_data`, `site_sle`, `vbeacon`, `wifi-conn-raw`, `wifi-unconn-raw`, `zone`
*
*/
public Output> topics() {
return this.topics;
}
/**
* enum: `aws-sns`, `google-pubsub`, `http-post`, `oauth2`, `splunk`
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return enum: `aws-sns`, `google-pubsub`, `http-post`, `oauth2`, `splunk`
*
*/
public Output type() {
return this.type;
}
@Export(name="url", refs={String.class}, tree="[0]")
private Output url;
public Output url() {
return this.url;
}
/**
* when url uses HTTPS, whether to verify the certificate
*
*/
@Export(name="verifyCert", refs={Boolean.class}, tree="[0]")
private Output verifyCert;
/**
* @return when url uses HTTPS, whether to verify the certificate
*
*/
public Output verifyCert() {
return this.verifyCert;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Webhook(java.lang.String name) {
this(name, WebhookArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Webhook(java.lang.String name, WebhookArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Webhook(java.lang.String name, WebhookArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("junipermist:site/webhook:Webhook", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Webhook(java.lang.String name, Output id, @Nullable WebhookState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("junipermist:site/webhook:Webhook", name, state, makeResourceOptions(options, id), false);
}
private static WebhookArgs makeArgs(WebhookArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? WebhookArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"oauth2ClientSecret",
"oauth2Password",
"secret",
"splunkToken"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Webhook get(java.lang.String name, Output id, @Nullable WebhookState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Webhook(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy