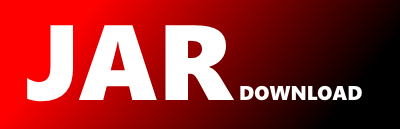
com.pulumi.keycloak.RealmArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keycloak Show documentation
Show all versions of keycloak Show documentation
A Pulumi package for creating and managing keycloak cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.keycloak;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.keycloak.inputs.RealmInternationalizationArgs;
import com.pulumi.keycloak.inputs.RealmOtpPolicyArgs;
import com.pulumi.keycloak.inputs.RealmSecurityDefensesArgs;
import com.pulumi.keycloak.inputs.RealmSmtpServerArgs;
import com.pulumi.keycloak.inputs.RealmWebAuthnPasswordlessPolicyArgs;
import com.pulumi.keycloak.inputs.RealmWebAuthnPolicyArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RealmArgs extends com.pulumi.resources.ResourceArgs {
public static final RealmArgs Empty = new RealmArgs();
@Import(name="accessCodeLifespan")
private @Nullable Output accessCodeLifespan;
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy