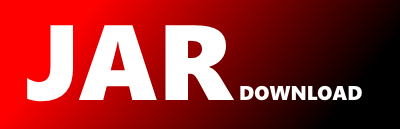
com.pulumi.keycloak.ldap.FullNameMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keycloak Show documentation
Show all versions of keycloak Show documentation
A Pulumi package for creating and managing keycloak cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.keycloak.ldap;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.keycloak.Utilities;
import com.pulumi.keycloak.ldap.FullNameMapperArgs;
import com.pulumi.keycloak.ldap.inputs.FullNameMapperState;
import java.lang.Boolean;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## # keycloak.ldap.FullNameMapper
*
* Allows for creating and managing full name mappers for Keycloak users federated
* via LDAP.
*
* The LDAP full name mapper can map a user's full name from an LDAP attribute
* to the first and last name attributes of a Keycloak user.
*
* ### Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.keycloak.Realm;
* import com.pulumi.keycloak.RealmArgs;
* import com.pulumi.keycloak.ldap.UserFederation;
* import com.pulumi.keycloak.ldap.UserFederationArgs;
* import com.pulumi.keycloak.ldap.FullNameMapper;
* import com.pulumi.keycloak.ldap.FullNameMapperArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var realm = new Realm("realm", RealmArgs.builder()
* .realm("test")
* .enabled(true)
* .build());
*
* var ldapUserFederation = new UserFederation("ldapUserFederation", UserFederationArgs.builder()
* .name("openldap")
* .realmId(realm.id())
* .usernameLdapAttribute("cn")
* .rdnLdapAttribute("cn")
* .uuidLdapAttribute("entryDN")
* .userObjectClasses(
* "simpleSecurityObject",
* "organizationalRole")
* .connectionUrl("ldap://openldap")
* .usersDn("dc=example,dc=org")
* .bindDn("cn=admin,dc=example,dc=org")
* .bindCredential("admin")
* .build());
*
* var ldapFullNameMapper = new FullNameMapper("ldapFullNameMapper", FullNameMapperArgs.builder()
* .realmId(realm.id())
* .ldapUserFederationId(ldapUserFederation.id())
* .name("full-name-mapper")
* .ldapFullNameAttribute("cn")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Argument Reference
*
* The following arguments are supported:
*
* - `realm_id` - (Required) The realm that this LDAP mapper will exist in.
* - `ldap_user_federation_id` - (Required) The ID of the LDAP user federation provider to attach this mapper to.
* - `name` - (Required) Display name of this mapper when displayed in the console.
* - `ldap_full_name_attribute` - (Required) The name of the LDAP attribute containing the user's full name.
* - `read_only` - (Optional) When `true`, updates to a user within Keycloak will not be written back to LDAP. Defaults to `false`.
* - `write_only` - (Optional) When `true`, this mapper will only be used to write updates to LDAP. Defaults to `false`.
*
* ### Import
*
* LDAP mappers can be imported using the format `{{realm_id}}/{{ldap_user_federation_id}}/{{ldap_mapper_id}}`.
* The ID of the LDAP user federation provider and the mapper can be found within
* the Keycloak GUI, and they are typically GUIDs:
*
*/
@ResourceType(type="keycloak:ldap/fullNameMapper:FullNameMapper")
public class FullNameMapper extends com.pulumi.resources.CustomResource {
@Export(name="ldapFullNameAttribute", refs={String.class}, tree="[0]")
private Output ldapFullNameAttribute;
public Output ldapFullNameAttribute() {
return this.ldapFullNameAttribute;
}
/**
* The ldap user federation provider to attach this mapper to.
*
*/
@Export(name="ldapUserFederationId", refs={String.class}, tree="[0]")
private Output ldapUserFederationId;
/**
* @return The ldap user federation provider to attach this mapper to.
*
*/
public Output ldapUserFederationId() {
return this.ldapUserFederationId;
}
/**
* Display name of the mapper when displayed in the console.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Display name of the mapper when displayed in the console.
*
*/
public Output name() {
return this.name;
}
@Export(name="readOnly", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> readOnly;
public Output> readOnly() {
return Codegen.optional(this.readOnly);
}
/**
* The realm in which the ldap user federation provider exists.
*
*/
@Export(name="realmId", refs={String.class}, tree="[0]")
private Output realmId;
/**
* @return The realm in which the ldap user federation provider exists.
*
*/
public Output realmId() {
return this.realmId;
}
@Export(name="writeOnly", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> writeOnly;
public Output> writeOnly() {
return Codegen.optional(this.writeOnly);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public FullNameMapper(java.lang.String name) {
this(name, FullNameMapperArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public FullNameMapper(java.lang.String name, FullNameMapperArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public FullNameMapper(java.lang.String name, FullNameMapperArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("keycloak:ldap/fullNameMapper:FullNameMapper", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private FullNameMapper(java.lang.String name, Output id, @Nullable FullNameMapperState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("keycloak:ldap/fullNameMapper:FullNameMapper", name, state, makeResourceOptions(options, id), false);
}
private static FullNameMapperArgs makeArgs(FullNameMapperArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? FullNameMapperArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static FullNameMapper get(java.lang.String name, Output id, @Nullable FullNameMapperState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new FullNameMapper(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy