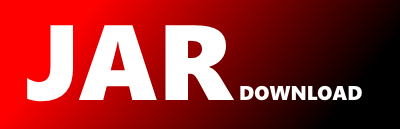
com.pulumi.keycloak.AttributeImporterIdentityProviderMapperArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keycloak Show documentation
Show all versions of keycloak Show documentation
A Pulumi package for creating and managing keycloak cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.keycloak;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AttributeImporterIdentityProviderMapperArgs extends com.pulumi.resources.ResourceArgs {
public static final AttributeImporterIdentityProviderMapperArgs Empty = new AttributeImporterIdentityProviderMapperArgs();
/**
* For SAML based providers, this is the friendly name of the attribute to search for in the assertion. Conflicts with `attribute_name`.
*
*/
@Import(name="attributeFriendlyName")
private @Nullable Output attributeFriendlyName;
/**
* @return For SAML based providers, this is the friendly name of the attribute to search for in the assertion. Conflicts with `attribute_name`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy