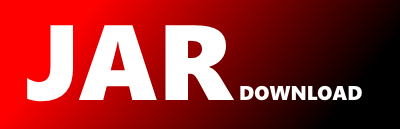
com.pulumi.keycloak.ProviderArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.keycloak;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ProviderArgs extends com.pulumi.resources.ResourceArgs {
public static final ProviderArgs Empty = new ProviderArgs();
@Import(name="additionalHeaders", json=true)
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy