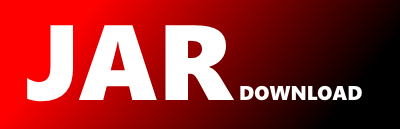
com.pulumi.keycloak.inputs.RealmUserProfileAttributeArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of keycloak Show documentation
Show all versions of keycloak Show documentation
A Pulumi package for creating and managing keycloak cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.keycloak.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.keycloak.inputs.RealmUserProfileAttributePermissionsArgs;
import com.pulumi.keycloak.inputs.RealmUserProfileAttributeValidatorArgs;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RealmUserProfileAttributeArgs extends com.pulumi.resources.ResourceArgs {
public static final RealmUserProfileAttributeArgs Empty = new RealmUserProfileAttributeArgs();
@Import(name="annotations")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy