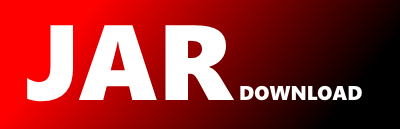
com.pulumi.kubernetes.policy.v1beta1.inputs.PodDisruptionBudgetStatusArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.policy.v1beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* PodDisruptionBudgetStatus represents information about the status of a PodDisruptionBudget. Status may trail the actual state of a system.
*
*/
public final class PodDisruptionBudgetStatusArgs extends com.pulumi.resources.ResourceArgs {
public static final PodDisruptionBudgetStatusArgs Empty = new PodDisruptionBudgetStatusArgs();
/**
* current number of healthy pods
*
*/
@Import(name="currentHealthy", required=true)
private Output currentHealthy;
/**
* @return current number of healthy pods
*
*/
public Output currentHealthy() {
return this.currentHealthy;
}
/**
* minimum desired number of healthy pods
*
*/
@Import(name="desiredHealthy", required=true)
private Output desiredHealthy;
/**
* @return minimum desired number of healthy pods
*
*/
public Output desiredHealthy() {
return this.desiredHealthy;
}
/**
* DisruptedPods contains information about pods whose eviction was processed by the API server eviction subresource handler but has not yet been observed by the PodDisruptionBudget controller. A pod will be in this map from the time when the API server processed the eviction request to the time when the pod is seen by PDB controller as having been marked for deletion (or after a timeout). The key in the map is the name of the pod and the value is the time when the API server processed the eviction request. If the deletion didn't occur and a pod is still there it will be removed from the list automatically by PodDisruptionBudget controller after some time. If everything goes smooth this map should be empty for the most of the time. Large number of entries in the map may indicate problems with pod deletions.
*
*/
@Import(name="disruptedPods")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy