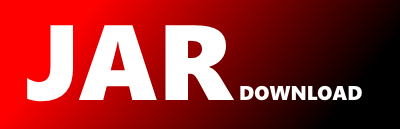
com.pulumi.kubernetes.discovery.v1.inputs.EndpointArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.discovery.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.core.v1.inputs.ObjectReferenceArgs;
import com.pulumi.kubernetes.discovery.v1.inputs.EndpointConditionsArgs;
import com.pulumi.kubernetes.discovery.v1.inputs.EndpointHintsArgs;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Endpoint represents a single logical "backend" implementing a service.
*
*/
public final class EndpointArgs extends com.pulumi.resources.ResourceArgs {
public static final EndpointArgs Empty = new EndpointArgs();
/**
* addresses of this endpoint. The contents of this field are interpreted according to the corresponding EndpointSlice addressType field. Consumers must handle different types of addresses in the context of their own capabilities. This must contain at least one address but no more than 100. These are all assumed to be fungible and clients may choose to only use the first element. Refer to: https://issue.k8s.io/106267
*
*/
@Import(name="addresses", required=true)
private Output> addresses;
/**
* @return addresses of this endpoint. The contents of this field are interpreted according to the corresponding EndpointSlice addressType field. Consumers must handle different types of addresses in the context of their own capabilities. This must contain at least one address but no more than 100. These are all assumed to be fungible and clients may choose to only use the first element. Refer to: https://issue.k8s.io/106267
*
*/
public Output> addresses() {
return this.addresses;
}
/**
* conditions contains information about the current status of the endpoint.
*
*/
@Import(name="conditions")
private @Nullable Output conditions;
/**
* @return conditions contains information about the current status of the endpoint.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy