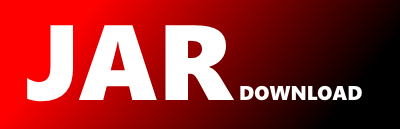
com.pulumi.kubernetes.apps.v1beta1.outputs.DeploymentSpecPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.apps.v1beta1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.kubernetes.apps.v1beta1.outputs.DeploymentStrategyPatch;
import com.pulumi.kubernetes.apps.v1beta1.outputs.RollbackConfigPatch;
import com.pulumi.kubernetes.core.v1.outputs.PodTemplateSpecPatch;
import com.pulumi.kubernetes.meta.v1.outputs.LabelSelectorPatch;
import java.lang.Boolean;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DeploymentSpecPatch {
/**
* @return Minimum number of seconds for which a newly created pod should be ready without any of its container crashing, for it to be considered available. Defaults to 0 (pod will be considered available as soon as it is ready)
*
*/
private @Nullable Integer minReadySeconds;
/**
* @return Indicates that the deployment is paused.
*
*/
private @Nullable Boolean paused;
/**
* @return The maximum time in seconds for a deployment to make progress before it is considered to be failed. The deployment controller will continue to process failed deployments and a condition with a ProgressDeadlineExceeded reason will be surfaced in the deployment status. Note that progress will not be estimated during the time a deployment is paused. Defaults to 600s.
*
*/
private @Nullable Integer progressDeadlineSeconds;
/**
* @return Number of desired pods. This is a pointer to distinguish between explicit zero and not specified. Defaults to 1.
*
*/
private @Nullable Integer replicas;
/**
* @return The number of old ReplicaSets to retain to allow rollback. This is a pointer to distinguish between explicit zero and not specified. Defaults to 2.
*
*/
private @Nullable Integer revisionHistoryLimit;
/**
* @return DEPRECATED. The config this deployment is rolling back to. Will be cleared after rollback is done.
*
*/
private @Nullable RollbackConfigPatch rollbackTo;
/**
* @return Label selector for pods. Existing ReplicaSets whose pods are selected by this will be the ones affected by this deployment.
*
*/
private @Nullable LabelSelectorPatch selector;
/**
* @return The deployment strategy to use to replace existing pods with new ones.
*
*/
private @Nullable DeploymentStrategyPatch strategy;
/**
* @return Template describes the pods that will be created.
*
*/
private @Nullable PodTemplateSpecPatch template;
private DeploymentSpecPatch() {}
/**
* @return Minimum number of seconds for which a newly created pod should be ready without any of its container crashing, for it to be considered available. Defaults to 0 (pod will be considered available as soon as it is ready)
*
*/
public Optional minReadySeconds() {
return Optional.ofNullable(this.minReadySeconds);
}
/**
* @return Indicates that the deployment is paused.
*
*/
public Optional paused() {
return Optional.ofNullable(this.paused);
}
/**
* @return The maximum time in seconds for a deployment to make progress before it is considered to be failed. The deployment controller will continue to process failed deployments and a condition with a ProgressDeadlineExceeded reason will be surfaced in the deployment status. Note that progress will not be estimated during the time a deployment is paused. Defaults to 600s.
*
*/
public Optional progressDeadlineSeconds() {
return Optional.ofNullable(this.progressDeadlineSeconds);
}
/**
* @return Number of desired pods. This is a pointer to distinguish between explicit zero and not specified. Defaults to 1.
*
*/
public Optional replicas() {
return Optional.ofNullable(this.replicas);
}
/**
* @return The number of old ReplicaSets to retain to allow rollback. This is a pointer to distinguish between explicit zero and not specified. Defaults to 2.
*
*/
public Optional revisionHistoryLimit() {
return Optional.ofNullable(this.revisionHistoryLimit);
}
/**
* @return DEPRECATED. The config this deployment is rolling back to. Will be cleared after rollback is done.
*
*/
public Optional rollbackTo() {
return Optional.ofNullable(this.rollbackTo);
}
/**
* @return Label selector for pods. Existing ReplicaSets whose pods are selected by this will be the ones affected by this deployment.
*
*/
public Optional selector() {
return Optional.ofNullable(this.selector);
}
/**
* @return The deployment strategy to use to replace existing pods with new ones.
*
*/
public Optional strategy() {
return Optional.ofNullable(this.strategy);
}
/**
* @return Template describes the pods that will be created.
*
*/
public Optional template() {
return Optional.ofNullable(this.template);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DeploymentSpecPatch defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer minReadySeconds;
private @Nullable Boolean paused;
private @Nullable Integer progressDeadlineSeconds;
private @Nullable Integer replicas;
private @Nullable Integer revisionHistoryLimit;
private @Nullable RollbackConfigPatch rollbackTo;
private @Nullable LabelSelectorPatch selector;
private @Nullable DeploymentStrategyPatch strategy;
private @Nullable PodTemplateSpecPatch template;
public Builder() {}
public Builder(DeploymentSpecPatch defaults) {
Objects.requireNonNull(defaults);
this.minReadySeconds = defaults.minReadySeconds;
this.paused = defaults.paused;
this.progressDeadlineSeconds = defaults.progressDeadlineSeconds;
this.replicas = defaults.replicas;
this.revisionHistoryLimit = defaults.revisionHistoryLimit;
this.rollbackTo = defaults.rollbackTo;
this.selector = defaults.selector;
this.strategy = defaults.strategy;
this.template = defaults.template;
}
@CustomType.Setter
public Builder minReadySeconds(@Nullable Integer minReadySeconds) {
this.minReadySeconds = minReadySeconds;
return this;
}
@CustomType.Setter
public Builder paused(@Nullable Boolean paused) {
this.paused = paused;
return this;
}
@CustomType.Setter
public Builder progressDeadlineSeconds(@Nullable Integer progressDeadlineSeconds) {
this.progressDeadlineSeconds = progressDeadlineSeconds;
return this;
}
@CustomType.Setter
public Builder replicas(@Nullable Integer replicas) {
this.replicas = replicas;
return this;
}
@CustomType.Setter
public Builder revisionHistoryLimit(@Nullable Integer revisionHistoryLimit) {
this.revisionHistoryLimit = revisionHistoryLimit;
return this;
}
@CustomType.Setter
public Builder rollbackTo(@Nullable RollbackConfigPatch rollbackTo) {
this.rollbackTo = rollbackTo;
return this;
}
@CustomType.Setter
public Builder selector(@Nullable LabelSelectorPatch selector) {
this.selector = selector;
return this;
}
@CustomType.Setter
public Builder strategy(@Nullable DeploymentStrategyPatch strategy) {
this.strategy = strategy;
return this;
}
@CustomType.Setter
public Builder template(@Nullable PodTemplateSpecPatch template) {
this.template = template;
return this;
}
public DeploymentSpecPatch build() {
final var _resultValue = new DeploymentSpecPatch();
_resultValue.minReadySeconds = minReadySeconds;
_resultValue.paused = paused;
_resultValue.progressDeadlineSeconds = progressDeadlineSeconds;
_resultValue.replicas = replicas;
_resultValue.revisionHistoryLimit = revisionHistoryLimit;
_resultValue.rollbackTo = rollbackTo;
_resultValue.selector = selector;
_resultValue.strategy = strategy;
_resultValue.template = template;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy