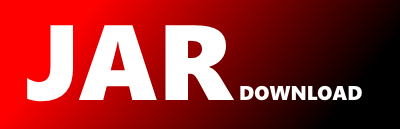
com.pulumi.kubernetes.extensions.v1beta1.outputs.RollingUpdateDeployment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.extensions.v1beta1.outputs;
import com.pulumi.core.Either;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class RollingUpdateDeployment {
/**
* @return The maximum number of pods that can be scheduled above the desired number of pods. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). This can not be 0 if MaxUnavailable is 0. Absolute number is calculated from percentage by rounding up. By default, a value of 1 is used. Example: when this is set to 30%, the new RC can be scaled up immediately when the rolling update starts, such that the total number of old and new pods do not exceed 130% of desired pods. Once old pods have been killed, new RC can be scaled up further, ensuring that total number of pods running at any time during the update is at most 130% of desired pods.
*
*/
private @Nullable Either maxSurge;
/**
* @return The maximum number of pods that can be unavailable during the update. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). Absolute number is calculated from percentage by rounding down. This can not be 0 if MaxSurge is 0. By default, a fixed value of 1 is used. Example: when this is set to 30%, the old RC can be scaled down to 70% of desired pods immediately when the rolling update starts. Once new pods are ready, old RC can be scaled down further, followed by scaling up the new RC, ensuring that the total number of pods available at all times during the update is at least 70% of desired pods.
*
*/
private @Nullable Either maxUnavailable;
private RollingUpdateDeployment() {}
/**
* @return The maximum number of pods that can be scheduled above the desired number of pods. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). This can not be 0 if MaxUnavailable is 0. Absolute number is calculated from percentage by rounding up. By default, a value of 1 is used. Example: when this is set to 30%, the new RC can be scaled up immediately when the rolling update starts, such that the total number of old and new pods do not exceed 130% of desired pods. Once old pods have been killed, new RC can be scaled up further, ensuring that total number of pods running at any time during the update is at most 130% of desired pods.
*
*/
public Optional> maxSurge() {
return Optional.ofNullable(this.maxSurge);
}
/**
* @return The maximum number of pods that can be unavailable during the update. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). Absolute number is calculated from percentage by rounding down. This can not be 0 if MaxSurge is 0. By default, a fixed value of 1 is used. Example: when this is set to 30%, the old RC can be scaled down to 70% of desired pods immediately when the rolling update starts. Once new pods are ready, old RC can be scaled down further, followed by scaling up the new RC, ensuring that the total number of pods available at all times during the update is at least 70% of desired pods.
*
*/
public Optional> maxUnavailable() {
return Optional.ofNullable(this.maxUnavailable);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(RollingUpdateDeployment defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Either maxSurge;
private @Nullable Either maxUnavailable;
public Builder() {}
public Builder(RollingUpdateDeployment defaults) {
Objects.requireNonNull(defaults);
this.maxSurge = defaults.maxSurge;
this.maxUnavailable = defaults.maxUnavailable;
}
@CustomType.Setter
public Builder maxSurge(@Nullable Either maxSurge) {
this.maxSurge = maxSurge;
return this;
}
@CustomType.Setter
public Builder maxUnavailable(@Nullable Either maxUnavailable) {
this.maxUnavailable = maxUnavailable;
return this;
}
public RollingUpdateDeployment build() {
final var _resultValue = new RollingUpdateDeployment();
_resultValue.maxSurge = maxSurge;
_resultValue.maxUnavailable = maxUnavailable;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy