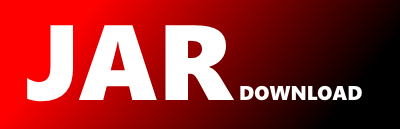
com.pulumi.kubernetes.meta.v1.inputs.StatusDetailsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.meta.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.kubernetes.meta.v1.inputs.StatusCauseArgs;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* StatusDetails is a set of additional properties that MAY be set by the server to provide additional information about a response. The Reason field of a Status object defines what attributes will be set. Clients must ignore fields that do not match the defined type of each attribute, and should assume that any attribute may be empty, invalid, or under defined.
*
*/
public final class StatusDetailsArgs extends com.pulumi.resources.ResourceArgs {
public static final StatusDetailsArgs Empty = new StatusDetailsArgs();
/**
* The Causes array includes more details associated with the StatusReason failure. Not all StatusReasons may provide detailed causes.
*
*/
@Import(name="causes")
private @Nullable Output> causes;
/**
* @return The Causes array includes more details associated with the StatusReason failure. Not all StatusReasons may provide detailed causes.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy