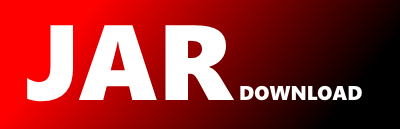
com.pulumi.kubernetes.meta.v1.outputs.ConditionPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.meta.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ConditionPatch {
/**
* @return lastTransitionTime is the last time the condition transitioned from one status to another. This should be when the underlying condition changed. If that is not known, then using the time when the API field changed is acceptable.
*
*/
private @Nullable String lastTransitionTime;
/**
* @return message is a human readable message indicating details about the transition. This may be an empty string.
*
*/
private @Nullable String message;
/**
* @return observedGeneration represents the .metadata.generation that the condition was set based upon. For instance, if .metadata.generation is currently 12, but the .status.conditions[x].observedGeneration is 9, the condition is out of date with respect to the current state of the instance.
*
*/
private @Nullable Integer observedGeneration;
/**
* @return reason contains a programmatic identifier indicating the reason for the condition's last transition. Producers of specific condition types may define expected values and meanings for this field, and whether the values are considered a guaranteed API. The value should be a CamelCase string. This field may not be empty.
*
*/
private @Nullable String reason;
/**
* @return status of the condition, one of True, False, Unknown.
*
*/
private @Nullable String status;
/**
* @return type of condition in CamelCase or in foo.example.com/CamelCase.
*
*/
private @Nullable String type;
private ConditionPatch() {}
/**
* @return lastTransitionTime is the last time the condition transitioned from one status to another. This should be when the underlying condition changed. If that is not known, then using the time when the API field changed is acceptable.
*
*/
public Optional lastTransitionTime() {
return Optional.ofNullable(this.lastTransitionTime);
}
/**
* @return message is a human readable message indicating details about the transition. This may be an empty string.
*
*/
public Optional message() {
return Optional.ofNullable(this.message);
}
/**
* @return observedGeneration represents the .metadata.generation that the condition was set based upon. For instance, if .metadata.generation is currently 12, but the .status.conditions[x].observedGeneration is 9, the condition is out of date with respect to the current state of the instance.
*
*/
public Optional observedGeneration() {
return Optional.ofNullable(this.observedGeneration);
}
/**
* @return reason contains a programmatic identifier indicating the reason for the condition's last transition. Producers of specific condition types may define expected values and meanings for this field, and whether the values are considered a guaranteed API. The value should be a CamelCase string. This field may not be empty.
*
*/
public Optional reason() {
return Optional.ofNullable(this.reason);
}
/**
* @return status of the condition, one of True, False, Unknown.
*
*/
public Optional status() {
return Optional.ofNullable(this.status);
}
/**
* @return type of condition in CamelCase or in foo.example.com/CamelCase.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ConditionPatch defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String lastTransitionTime;
private @Nullable String message;
private @Nullable Integer observedGeneration;
private @Nullable String reason;
private @Nullable String status;
private @Nullable String type;
public Builder() {}
public Builder(ConditionPatch defaults) {
Objects.requireNonNull(defaults);
this.lastTransitionTime = defaults.lastTransitionTime;
this.message = defaults.message;
this.observedGeneration = defaults.observedGeneration;
this.reason = defaults.reason;
this.status = defaults.status;
this.type = defaults.type;
}
@CustomType.Setter
public Builder lastTransitionTime(@Nullable String lastTransitionTime) {
this.lastTransitionTime = lastTransitionTime;
return this;
}
@CustomType.Setter
public Builder message(@Nullable String message) {
this.message = message;
return this;
}
@CustomType.Setter
public Builder observedGeneration(@Nullable Integer observedGeneration) {
this.observedGeneration = observedGeneration;
return this;
}
@CustomType.Setter
public Builder reason(@Nullable String reason) {
this.reason = reason;
return this;
}
@CustomType.Setter
public Builder status(@Nullable String status) {
this.status = status;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
public ConditionPatch build() {
final var _resultValue = new ConditionPatch();
_resultValue.lastTransitionTime = lastTransitionTime;
_resultValue.message = message;
_resultValue.observedGeneration = observedGeneration;
_resultValue.reason = reason;
_resultValue.status = status;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy