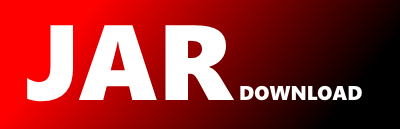
com.pulumi.kubernetes.events.v1beta1.EventArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.events.v1beta1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.core.v1.inputs.EventSourceArgs;
import com.pulumi.kubernetes.core.v1.inputs.ObjectReferenceArgs;
import com.pulumi.kubernetes.events.v1beta1.inputs.EventSeriesArgs;
import com.pulumi.kubernetes.meta.v1.inputs.ObjectMetaArgs;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EventArgs extends com.pulumi.resources.ResourceArgs {
public static final EventArgs Empty = new EventArgs();
/**
* What action was taken/failed regarding to the regarding object.
*
*/
@Import(name="action")
private @Nullable Output action;
/**
* @return What action was taken/failed regarding to the regarding object.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy