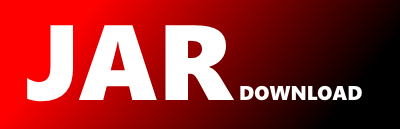
com.pulumi.kubernetes.extensions.v1beta1.inputs.HTTPIngressPathArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.extensions.v1beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.extensions.v1beta1.inputs.IngressBackendArgs;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* HTTPIngressPath associates a path regex with a backend. Incoming urls matching the path are forwarded to the backend.
*
*/
public final class HTTPIngressPathArgs extends com.pulumi.resources.ResourceArgs {
public static final HTTPIngressPathArgs Empty = new HTTPIngressPathArgs();
/**
* Backend defines the referenced service endpoint to which the traffic will be forwarded to.
*
*/
@Import(name="backend", required=true)
private Output backend;
/**
* @return Backend defines the referenced service endpoint to which the traffic will be forwarded to.
*
*/
public Output backend() {
return this.backend;
}
/**
* Path is an extended POSIX regex as defined by IEEE Std 1003.1, (i.e this follows the egrep/unix syntax, not the perl syntax) matched against the path of an incoming request. Currently it can contain characters disallowed from the conventional "path" part of a URL as defined by RFC 3986. Paths must begin with a '/'. If unspecified, the path defaults to a catch all sending traffic to the backend.
*
*/
@Import(name="path")
private @Nullable Output path;
/**
* @return Path is an extended POSIX regex as defined by IEEE Std 1003.1, (i.e this follows the egrep/unix syntax, not the perl syntax) matched against the path of an incoming request. Currently it can contain characters disallowed from the conventional "path" part of a URL as defined by RFC 3986. Paths must begin with a '/'. If unspecified, the path defaults to a catch all sending traffic to the backend.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy