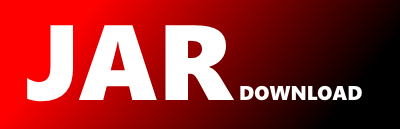
com.pulumi.kubernetes.policy.v1.outputs.PodDisruptionBudgetStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.policy.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.meta.v1.outputs.Condition;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class PodDisruptionBudgetStatus {
/**
* @return Conditions contain conditions for PDB. The disruption controller sets the DisruptionAllowed condition. The following are known values for the reason field (additional reasons could be added in the future): - SyncFailed: The controller encountered an error and wasn't able to compute
* the number of allowed disruptions. Therefore no disruptions are
* allowed and the status of the condition will be False.
* - InsufficientPods: The number of pods are either at or below the number
* required by the PodDisruptionBudget. No disruptions are
* allowed and the status of the condition will be False.
* - SufficientPods: There are more pods than required by the PodDisruptionBudget.
* The condition will be True, and the number of allowed
* disruptions are provided by the disruptionsAllowed property.
*
*/
private @Nullable List conditions;
/**
* @return current number of healthy pods
*
*/
private Integer currentHealthy;
/**
* @return minimum desired number of healthy pods
*
*/
private Integer desiredHealthy;
/**
* @return DisruptedPods contains information about pods whose eviction was processed by the API server eviction subresource handler but has not yet been observed by the PodDisruptionBudget controller. A pod will be in this map from the time when the API server processed the eviction request to the time when the pod is seen by PDB controller as having been marked for deletion (or after a timeout). The key in the map is the name of the pod and the value is the time when the API server processed the eviction request. If the deletion didn't occur and a pod is still there it will be removed from the list automatically by PodDisruptionBudget controller after some time. If everything goes smooth this map should be empty for the most of the time. Large number of entries in the map may indicate problems with pod deletions.
*
*/
private @Nullable Map disruptedPods;
/**
* @return Number of pod disruptions that are currently allowed.
*
*/
private Integer disruptionsAllowed;
/**
* @return total number of pods counted by this disruption budget
*
*/
private Integer expectedPods;
/**
* @return Most recent generation observed when updating this PDB status. DisruptionsAllowed and other status information is valid only if observedGeneration equals to PDB's object generation.
*
*/
private @Nullable Integer observedGeneration;
private PodDisruptionBudgetStatus() {}
/**
* @return Conditions contain conditions for PDB. The disruption controller sets the DisruptionAllowed condition. The following are known values for the reason field (additional reasons could be added in the future): - SyncFailed: The controller encountered an error and wasn't able to compute
* the number of allowed disruptions. Therefore no disruptions are
* allowed and the status of the condition will be False.
* - InsufficientPods: The number of pods are either at or below the number
* required by the PodDisruptionBudget. No disruptions are
* allowed and the status of the condition will be False.
* - SufficientPods: There are more pods than required by the PodDisruptionBudget.
* The condition will be True, and the number of allowed
* disruptions are provided by the disruptionsAllowed property.
*
*/
public List conditions() {
return this.conditions == null ? List.of() : this.conditions;
}
/**
* @return current number of healthy pods
*
*/
public Integer currentHealthy() {
return this.currentHealthy;
}
/**
* @return minimum desired number of healthy pods
*
*/
public Integer desiredHealthy() {
return this.desiredHealthy;
}
/**
* @return DisruptedPods contains information about pods whose eviction was processed by the API server eviction subresource handler but has not yet been observed by the PodDisruptionBudget controller. A pod will be in this map from the time when the API server processed the eviction request to the time when the pod is seen by PDB controller as having been marked for deletion (or after a timeout). The key in the map is the name of the pod and the value is the time when the API server processed the eviction request. If the deletion didn't occur and a pod is still there it will be removed from the list automatically by PodDisruptionBudget controller after some time. If everything goes smooth this map should be empty for the most of the time. Large number of entries in the map may indicate problems with pod deletions.
*
*/
public Map disruptedPods() {
return this.disruptedPods == null ? Map.of() : this.disruptedPods;
}
/**
* @return Number of pod disruptions that are currently allowed.
*
*/
public Integer disruptionsAllowed() {
return this.disruptionsAllowed;
}
/**
* @return total number of pods counted by this disruption budget
*
*/
public Integer expectedPods() {
return this.expectedPods;
}
/**
* @return Most recent generation observed when updating this PDB status. DisruptionsAllowed and other status information is valid only if observedGeneration equals to PDB's object generation.
*
*/
public Optional observedGeneration() {
return Optional.ofNullable(this.observedGeneration);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PodDisruptionBudgetStatus defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List conditions;
private Integer currentHealthy;
private Integer desiredHealthy;
private @Nullable Map disruptedPods;
private Integer disruptionsAllowed;
private Integer expectedPods;
private @Nullable Integer observedGeneration;
public Builder() {}
public Builder(PodDisruptionBudgetStatus defaults) {
Objects.requireNonNull(defaults);
this.conditions = defaults.conditions;
this.currentHealthy = defaults.currentHealthy;
this.desiredHealthy = defaults.desiredHealthy;
this.disruptedPods = defaults.disruptedPods;
this.disruptionsAllowed = defaults.disruptionsAllowed;
this.expectedPods = defaults.expectedPods;
this.observedGeneration = defaults.observedGeneration;
}
@CustomType.Setter
public Builder conditions(@Nullable List conditions) {
this.conditions = conditions;
return this;
}
public Builder conditions(Condition... conditions) {
return conditions(List.of(conditions));
}
@CustomType.Setter
public Builder currentHealthy(Integer currentHealthy) {
if (currentHealthy == null) {
throw new MissingRequiredPropertyException("PodDisruptionBudgetStatus", "currentHealthy");
}
this.currentHealthy = currentHealthy;
return this;
}
@CustomType.Setter
public Builder desiredHealthy(Integer desiredHealthy) {
if (desiredHealthy == null) {
throw new MissingRequiredPropertyException("PodDisruptionBudgetStatus", "desiredHealthy");
}
this.desiredHealthy = desiredHealthy;
return this;
}
@CustomType.Setter
public Builder disruptedPods(@Nullable Map disruptedPods) {
this.disruptedPods = disruptedPods;
return this;
}
@CustomType.Setter
public Builder disruptionsAllowed(Integer disruptionsAllowed) {
if (disruptionsAllowed == null) {
throw new MissingRequiredPropertyException("PodDisruptionBudgetStatus", "disruptionsAllowed");
}
this.disruptionsAllowed = disruptionsAllowed;
return this;
}
@CustomType.Setter
public Builder expectedPods(Integer expectedPods) {
if (expectedPods == null) {
throw new MissingRequiredPropertyException("PodDisruptionBudgetStatus", "expectedPods");
}
this.expectedPods = expectedPods;
return this;
}
@CustomType.Setter
public Builder observedGeneration(@Nullable Integer observedGeneration) {
this.observedGeneration = observedGeneration;
return this;
}
public PodDisruptionBudgetStatus build() {
final var _resultValue = new PodDisruptionBudgetStatus();
_resultValue.conditions = conditions;
_resultValue.currentHealthy = currentHealthy;
_resultValue.desiredHealthy = desiredHealthy;
_resultValue.disruptedPods = disruptedPods;
_resultValue.disruptionsAllowed = disruptionsAllowed;
_resultValue.expectedPods = expectedPods;
_resultValue.observedGeneration = observedGeneration;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy