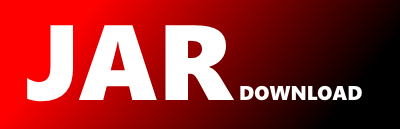
com.pulumi.kubernetes.apiextensions.v1.outputs.CustomResourceColumnDefinitionPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.apiextensions.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CustomResourceColumnDefinitionPatch {
/**
* @return description is a human readable description of this column.
*
*/
private @Nullable String description;
/**
* @return format is an optional OpenAPI type definition for this column. The 'name' format is applied to the primary identifier column to assist in clients identifying column is the resource name. See https://github.com/OAI/OpenAPI-Specification/blob/master/versions/2.0.md#data-types for details.
*
*/
private @Nullable String format;
/**
* @return jsonPath is a simple JSON path (i.e. with array notation) which is evaluated against each custom resource to produce the value for this column.
*
*/
private @Nullable String jsonPath;
/**
* @return name is a human readable name for the column.
*
*/
private @Nullable String name;
/**
* @return priority is an integer defining the relative importance of this column compared to others. Lower numbers are considered higher priority. Columns that may be omitted in limited space scenarios should be given a priority greater than 0.
*
*/
private @Nullable Integer priority;
/**
* @return type is an OpenAPI type definition for this column. See https://github.com/OAI/OpenAPI-Specification/blob/master/versions/2.0.md#data-types for details.
*
*/
private @Nullable String type;
private CustomResourceColumnDefinitionPatch() {}
/**
* @return description is a human readable description of this column.
*
*/
public Optional description() {
return Optional.ofNullable(this.description);
}
/**
* @return format is an optional OpenAPI type definition for this column. The 'name' format is applied to the primary identifier column to assist in clients identifying column is the resource name. See https://github.com/OAI/OpenAPI-Specification/blob/master/versions/2.0.md#data-types for details.
*
*/
public Optional format() {
return Optional.ofNullable(this.format);
}
/**
* @return jsonPath is a simple JSON path (i.e. with array notation) which is evaluated against each custom resource to produce the value for this column.
*
*/
public Optional jsonPath() {
return Optional.ofNullable(this.jsonPath);
}
/**
* @return name is a human readable name for the column.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return priority is an integer defining the relative importance of this column compared to others. Lower numbers are considered higher priority. Columns that may be omitted in limited space scenarios should be given a priority greater than 0.
*
*/
public Optional priority() {
return Optional.ofNullable(this.priority);
}
/**
* @return type is an OpenAPI type definition for this column. See https://github.com/OAI/OpenAPI-Specification/blob/master/versions/2.0.md#data-types for details.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CustomResourceColumnDefinitionPatch defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String description;
private @Nullable String format;
private @Nullable String jsonPath;
private @Nullable String name;
private @Nullable Integer priority;
private @Nullable String type;
public Builder() {}
public Builder(CustomResourceColumnDefinitionPatch defaults) {
Objects.requireNonNull(defaults);
this.description = defaults.description;
this.format = defaults.format;
this.jsonPath = defaults.jsonPath;
this.name = defaults.name;
this.priority = defaults.priority;
this.type = defaults.type;
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder format(@Nullable String format) {
this.format = format;
return this;
}
@CustomType.Setter
public Builder jsonPath(@Nullable String jsonPath) {
this.jsonPath = jsonPath;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder priority(@Nullable Integer priority) {
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
public CustomResourceColumnDefinitionPatch build() {
final var _resultValue = new CustomResourceColumnDefinitionPatch();
_resultValue.description = description;
_resultValue.format = format;
_resultValue.jsonPath = jsonPath;
_resultValue.name = name;
_resultValue.priority = priority;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy