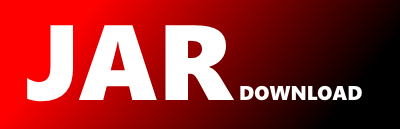
com.pulumi.kubernetes.apiextensions.v1.outputs.CustomResourceDefinitionSpec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.apiextensions.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.apiextensions.v1.outputs.CustomResourceConversion;
import com.pulumi.kubernetes.apiextensions.v1.outputs.CustomResourceDefinitionNames;
import com.pulumi.kubernetes.apiextensions.v1.outputs.CustomResourceDefinitionVersion;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CustomResourceDefinitionSpec {
/**
* @return conversion defines conversion settings for the CRD.
*
*/
private @Nullable CustomResourceConversion conversion;
/**
* @return group is the API group of the defined custom resource. The custom resources are served under `/apis/<group>/...`. Must match the name of the CustomResourceDefinition (in the form `<names.plural>.<group>`).
*
*/
private String group;
/**
* @return names specify the resource and kind names for the custom resource.
*
*/
private CustomResourceDefinitionNames names;
/**
* @return preserveUnknownFields indicates that object fields which are not specified in the OpenAPI schema should be preserved when persisting to storage. apiVersion, kind, metadata and known fields inside metadata are always preserved. This field is deprecated in favor of setting `x-preserve-unknown-fields` to true in `spec.versions[*].schema.openAPIV3Schema`. See https://kubernetes.io/docs/tasks/extend-kubernetes/custom-resources/custom-resource-definitions/#field-pruning for details.
*
*/
private @Nullable Boolean preserveUnknownFields;
/**
* @return scope indicates whether the defined custom resource is cluster- or namespace-scoped. Allowed values are `Cluster` and `Namespaced`.
*
*/
private String scope;
/**
* @return versions is the list of all API versions of the defined custom resource. Version names are used to compute the order in which served versions are listed in API discovery. If the version string is "kube-like", it will sort above non "kube-like" version strings, which are ordered lexicographically. "Kube-like" versions start with a "v", then are followed by a number (the major version), then optionally the string "alpha" or "beta" and another number (the minor version). These are sorted first by GA > beta > alpha (where GA is a version with no suffix such as beta or alpha), and then by comparing major version, then minor version. An example sorted list of versions: v10, v2, v1, v11beta2, v10beta3, v3beta1, v12alpha1, v11alpha2, foo1, foo10.
*
*/
private List versions;
private CustomResourceDefinitionSpec() {}
/**
* @return conversion defines conversion settings for the CRD.
*
*/
public Optional conversion() {
return Optional.ofNullable(this.conversion);
}
/**
* @return group is the API group of the defined custom resource. The custom resources are served under `/apis/<group>/...`. Must match the name of the CustomResourceDefinition (in the form `<names.plural>.<group>`).
*
*/
public String group() {
return this.group;
}
/**
* @return names specify the resource and kind names for the custom resource.
*
*/
public CustomResourceDefinitionNames names() {
return this.names;
}
/**
* @return preserveUnknownFields indicates that object fields which are not specified in the OpenAPI schema should be preserved when persisting to storage. apiVersion, kind, metadata and known fields inside metadata are always preserved. This field is deprecated in favor of setting `x-preserve-unknown-fields` to true in `spec.versions[*].schema.openAPIV3Schema`. See https://kubernetes.io/docs/tasks/extend-kubernetes/custom-resources/custom-resource-definitions/#field-pruning for details.
*
*/
public Optional preserveUnknownFields() {
return Optional.ofNullable(this.preserveUnknownFields);
}
/**
* @return scope indicates whether the defined custom resource is cluster- or namespace-scoped. Allowed values are `Cluster` and `Namespaced`.
*
*/
public String scope() {
return this.scope;
}
/**
* @return versions is the list of all API versions of the defined custom resource. Version names are used to compute the order in which served versions are listed in API discovery. If the version string is "kube-like", it will sort above non "kube-like" version strings, which are ordered lexicographically. "Kube-like" versions start with a "v", then are followed by a number (the major version), then optionally the string "alpha" or "beta" and another number (the minor version). These are sorted first by GA > beta > alpha (where GA is a version with no suffix such as beta or alpha), and then by comparing major version, then minor version. An example sorted list of versions: v10, v2, v1, v11beta2, v10beta3, v3beta1, v12alpha1, v11alpha2, foo1, foo10.
*
*/
public List versions() {
return this.versions;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CustomResourceDefinitionSpec defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable CustomResourceConversion conversion;
private String group;
private CustomResourceDefinitionNames names;
private @Nullable Boolean preserveUnknownFields;
private String scope;
private List versions;
public Builder() {}
public Builder(CustomResourceDefinitionSpec defaults) {
Objects.requireNonNull(defaults);
this.conversion = defaults.conversion;
this.group = defaults.group;
this.names = defaults.names;
this.preserveUnknownFields = defaults.preserveUnknownFields;
this.scope = defaults.scope;
this.versions = defaults.versions;
}
@CustomType.Setter
public Builder conversion(@Nullable CustomResourceConversion conversion) {
this.conversion = conversion;
return this;
}
@CustomType.Setter
public Builder group(String group) {
if (group == null) {
throw new MissingRequiredPropertyException("CustomResourceDefinitionSpec", "group");
}
this.group = group;
return this;
}
@CustomType.Setter
public Builder names(CustomResourceDefinitionNames names) {
if (names == null) {
throw new MissingRequiredPropertyException("CustomResourceDefinitionSpec", "names");
}
this.names = names;
return this;
}
@CustomType.Setter
public Builder preserveUnknownFields(@Nullable Boolean preserveUnknownFields) {
this.preserveUnknownFields = preserveUnknownFields;
return this;
}
@CustomType.Setter
public Builder scope(String scope) {
if (scope == null) {
throw new MissingRequiredPropertyException("CustomResourceDefinitionSpec", "scope");
}
this.scope = scope;
return this;
}
@CustomType.Setter
public Builder versions(List versions) {
if (versions == null) {
throw new MissingRequiredPropertyException("CustomResourceDefinitionSpec", "versions");
}
this.versions = versions;
return this;
}
public Builder versions(CustomResourceDefinitionVersion... versions) {
return versions(List.of(versions));
}
public CustomResourceDefinitionSpec build() {
final var _resultValue = new CustomResourceDefinitionSpec();
_resultValue.conversion = conversion;
_resultValue.group = group;
_resultValue.names = names;
_resultValue.preserveUnknownFields = preserveUnknownFields;
_resultValue.scope = scope;
_resultValue.versions = versions;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy