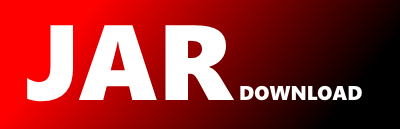
com.pulumi.kubernetes.autoscaling.v2.inputs.MetricSpecPatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.autoscaling.v2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.kubernetes.autoscaling.v2.inputs.ContainerResourceMetricSourcePatchArgs;
import com.pulumi.kubernetes.autoscaling.v2.inputs.ExternalMetricSourcePatchArgs;
import com.pulumi.kubernetes.autoscaling.v2.inputs.ObjectMetricSourcePatchArgs;
import com.pulumi.kubernetes.autoscaling.v2.inputs.PodsMetricSourcePatchArgs;
import com.pulumi.kubernetes.autoscaling.v2.inputs.ResourceMetricSourcePatchArgs;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* MetricSpec specifies how to scale based on a single metric (only `type` and one other matching field should be set at once).
*
*/
public final class MetricSpecPatchArgs extends com.pulumi.resources.ResourceArgs {
public static final MetricSpecPatchArgs Empty = new MetricSpecPatchArgs();
/**
* containerResource refers to a resource metric (such as those specified in requests and limits) known to Kubernetes describing a single container in each pod of the current scale target (e.g. CPU or memory). Such metrics are built in to Kubernetes, and have special scaling options on top of those available to normal per-pod metrics using the "pods" source. This is an alpha feature and can be enabled by the HPAContainerMetrics feature flag.
*
*/
@Import(name="containerResource")
private @Nullable Output containerResource;
/**
* @return containerResource refers to a resource metric (such as those specified in requests and limits) known to Kubernetes describing a single container in each pod of the current scale target (e.g. CPU or memory). Such metrics are built in to Kubernetes, and have special scaling options on top of those available to normal per-pod metrics using the "pods" source. This is an alpha feature and can be enabled by the HPAContainerMetrics feature flag.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy