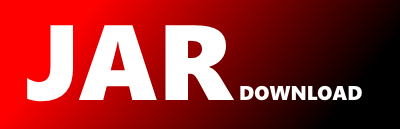
com.pulumi.kubernetes.resource.v1alpha2.inputs.NamedResourcesAttributePatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.resource.v1alpha2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.kubernetes.resource.v1alpha2.inputs.NamedResourcesIntSlicePatchArgs;
import com.pulumi.kubernetes.resource.v1alpha2.inputs.NamedResourcesStringSlicePatchArgs;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* NamedResourcesAttribute is a combination of an attribute name and its value.
*
*/
public final class NamedResourcesAttributePatchArgs extends com.pulumi.resources.ResourceArgs {
public static final NamedResourcesAttributePatchArgs Empty = new NamedResourcesAttributePatchArgs();
/**
* BoolValue is a true/false value.
*
*/
@Import(name="bool")
private @Nullable Output bool;
/**
* @return BoolValue is a true/false value.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy