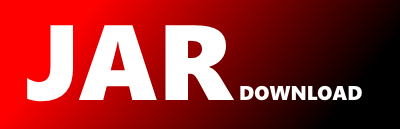
com.pulumi.kubernetes.resource.v1alpha2.inputs.ResourceClaimStatusArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.resource.v1alpha2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.kubernetes.resource.v1alpha2.inputs.AllocationResultArgs;
import com.pulumi.kubernetes.resource.v1alpha2.inputs.ResourceClaimConsumerReferenceArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ResourceClaimStatus tracks whether the resource has been allocated and what the resulting attributes are.
*
*/
public final class ResourceClaimStatusArgs extends com.pulumi.resources.ResourceArgs {
public static final ResourceClaimStatusArgs Empty = new ResourceClaimStatusArgs();
/**
* Allocation is set by the resource driver once a resource or set of resources has been allocated successfully. If this is not specified, the resources have not been allocated yet.
*
*/
@Import(name="allocation")
private @Nullable Output allocation;
/**
* @return Allocation is set by the resource driver once a resource or set of resources has been allocated successfully. If this is not specified, the resources have not been allocated yet.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy