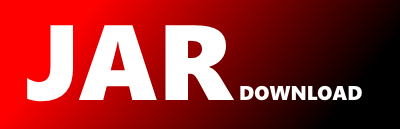
com.pulumi.kubernetes.coordination.v1.inputs.LeaseSpecPatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.coordination.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* LeaseSpec is a specification of a Lease.
*
*/
public final class LeaseSpecPatchArgs extends com.pulumi.resources.ResourceArgs {
public static final LeaseSpecPatchArgs Empty = new LeaseSpecPatchArgs();
/**
* acquireTime is a time when the current lease was acquired.
*
*/
@Import(name="acquireTime")
private @Nullable Output acquireTime;
/**
* @return acquireTime is a time when the current lease was acquired.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy