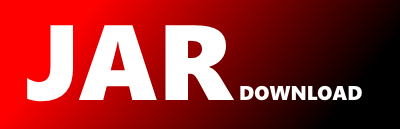
com.pulumi.kubernetes.extensions.v1beta1.inputs.DeploymentStatusArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.extensions.v1beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.kubernetes.extensions.v1beta1.inputs.DeploymentConditionArgs;
import java.lang.Integer;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* DeploymentStatus is the most recently observed status of the Deployment.
*
*/
public final class DeploymentStatusArgs extends com.pulumi.resources.ResourceArgs {
public static final DeploymentStatusArgs Empty = new DeploymentStatusArgs();
/**
* Total number of available pods (ready for at least minReadySeconds) targeted by this deployment.
*
*/
@Import(name="availableReplicas")
private @Nullable Output availableReplicas;
/**
* @return Total number of available pods (ready for at least minReadySeconds) targeted by this deployment.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy