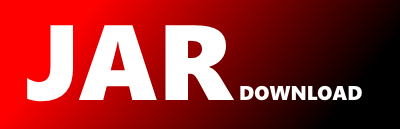
com.pulumi.kubernetes.storage.v1.inputs.VolumeAttachmentStatusArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.storage.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.storage.v1.inputs.VolumeErrorArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* VolumeAttachmentStatus is the status of a VolumeAttachment request.
*
*/
public final class VolumeAttachmentStatusArgs extends com.pulumi.resources.ResourceArgs {
public static final VolumeAttachmentStatusArgs Empty = new VolumeAttachmentStatusArgs();
/**
* attachError represents the last error encountered during attach operation, if any. This field must only be set by the entity completing the attach operation, i.e. the external-attacher.
*
*/
@Import(name="attachError")
private @Nullable Output attachError;
/**
* @return attachError represents the last error encountered during attach operation, if any. This field must only be set by the entity completing the attach operation, i.e. the external-attacher.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy