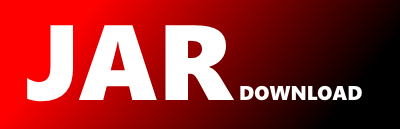
com.pulumi.kubernetes.storage.v1beta1.StorageClassArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.storage.v1beta1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.core.v1.inputs.TopologySelectorTermArgs;
import com.pulumi.kubernetes.meta.v1.inputs.ObjectMetaArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StorageClassArgs extends com.pulumi.resources.ResourceArgs {
public static final StorageClassArgs Empty = new StorageClassArgs();
/**
* AllowVolumeExpansion shows whether the storage class allow volume expand
*
*/
@Import(name="allowVolumeExpansion")
private @Nullable Output allowVolumeExpansion;
/**
* @return AllowVolumeExpansion shows whether the storage class allow volume expand
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy