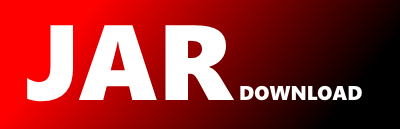
com.pulumi.kubernetes.resource.v1alpha3.outputs.DeviceRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.resource.v1alpha3.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.resource.v1alpha3.outputs.DeviceSelector;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DeviceRequest {
/**
* @return AdminAccess indicates that this is a claim for administrative access to the device(s). Claims with AdminAccess are expected to be used for monitoring or other management services for a device. They ignore all ordinary claims to the device with respect to access modes and any resource allocations.
*
*/
private @Nullable Boolean adminAccess;
/**
* @return AllocationMode and its related fields define how devices are allocated to satisfy this request. Supported values are:
*
* - ExactCount: This request is for a specific number of devices.
* This is the default. The exact number is provided in the
* count field.
*
* - All: This request is for all of the matching devices in a pool.
* Allocation will fail if some devices are already allocated,
* unless adminAccess is requested.
*
* If AlloctionMode is not specified, the default mode is ExactCount. If the mode is ExactCount and count is not specified, the default count is one. Any other requests must specify this field.
*
* More modes may get added in the future. Clients must refuse to handle requests with unknown modes.
*
*/
private @Nullable String allocationMode;
/**
* @return Count is used only when the count mode is "ExactCount". Must be greater than zero. If AllocationMode is ExactCount and this field is not specified, the default is one.
*
*/
private @Nullable Integer count;
/**
* @return DeviceClassName references a specific DeviceClass, which can define additional configuration and selectors to be inherited by this request.
*
* A class is required. Which classes are available depends on the cluster.
*
* Administrators may use this to restrict which devices may get requested by only installing classes with selectors for permitted devices. If users are free to request anything without restrictions, then administrators can create an empty DeviceClass for users to reference.
*
*/
private String deviceClassName;
/**
* @return Name can be used to reference this request in a pod.spec.containers[].resources.claims entry and in a constraint of the claim.
*
* Must be a DNS label.
*
*/
private String name;
/**
* @return Selectors define criteria which must be satisfied by a specific device in order for that device to be considered for this request. All selectors must be satisfied for a device to be considered.
*
*/
private @Nullable List selectors;
private DeviceRequest() {}
/**
* @return AdminAccess indicates that this is a claim for administrative access to the device(s). Claims with AdminAccess are expected to be used for monitoring or other management services for a device. They ignore all ordinary claims to the device with respect to access modes and any resource allocations.
*
*/
public Optional adminAccess() {
return Optional.ofNullable(this.adminAccess);
}
/**
* @return AllocationMode and its related fields define how devices are allocated to satisfy this request. Supported values are:
*
* - ExactCount: This request is for a specific number of devices.
* This is the default. The exact number is provided in the
* count field.
*
* - All: This request is for all of the matching devices in a pool.
* Allocation will fail if some devices are already allocated,
* unless adminAccess is requested.
*
* If AlloctionMode is not specified, the default mode is ExactCount. If the mode is ExactCount and count is not specified, the default count is one. Any other requests must specify this field.
*
* More modes may get added in the future. Clients must refuse to handle requests with unknown modes.
*
*/
public Optional allocationMode() {
return Optional.ofNullable(this.allocationMode);
}
/**
* @return Count is used only when the count mode is "ExactCount". Must be greater than zero. If AllocationMode is ExactCount and this field is not specified, the default is one.
*
*/
public Optional count() {
return Optional.ofNullable(this.count);
}
/**
* @return DeviceClassName references a specific DeviceClass, which can define additional configuration and selectors to be inherited by this request.
*
* A class is required. Which classes are available depends on the cluster.
*
* Administrators may use this to restrict which devices may get requested by only installing classes with selectors for permitted devices. If users are free to request anything without restrictions, then administrators can create an empty DeviceClass for users to reference.
*
*/
public String deviceClassName() {
return this.deviceClassName;
}
/**
* @return Name can be used to reference this request in a pod.spec.containers[].resources.claims entry and in a constraint of the claim.
*
* Must be a DNS label.
*
*/
public String name() {
return this.name;
}
/**
* @return Selectors define criteria which must be satisfied by a specific device in order for that device to be considered for this request. All selectors must be satisfied for a device to be considered.
*
*/
public List selectors() {
return this.selectors == null ? List.of() : this.selectors;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DeviceRequest defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean adminAccess;
private @Nullable String allocationMode;
private @Nullable Integer count;
private String deviceClassName;
private String name;
private @Nullable List selectors;
public Builder() {}
public Builder(DeviceRequest defaults) {
Objects.requireNonNull(defaults);
this.adminAccess = defaults.adminAccess;
this.allocationMode = defaults.allocationMode;
this.count = defaults.count;
this.deviceClassName = defaults.deviceClassName;
this.name = defaults.name;
this.selectors = defaults.selectors;
}
@CustomType.Setter
public Builder adminAccess(@Nullable Boolean adminAccess) {
this.adminAccess = adminAccess;
return this;
}
@CustomType.Setter
public Builder allocationMode(@Nullable String allocationMode) {
this.allocationMode = allocationMode;
return this;
}
@CustomType.Setter
public Builder count(@Nullable Integer count) {
this.count = count;
return this;
}
@CustomType.Setter
public Builder deviceClassName(String deviceClassName) {
if (deviceClassName == null) {
throw new MissingRequiredPropertyException("DeviceRequest", "deviceClassName");
}
this.deviceClassName = deviceClassName;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("DeviceRequest", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder selectors(@Nullable List selectors) {
this.selectors = selectors;
return this;
}
public Builder selectors(DeviceSelector... selectors) {
return selectors(List.of(selectors));
}
public DeviceRequest build() {
final var _resultValue = new DeviceRequest();
_resultValue.adminAccess = adminAccess;
_resultValue.allocationMode = allocationMode;
_resultValue.count = count;
_resultValue.deviceClassName = deviceClassName;
_resultValue.name = name;
_resultValue.selectors = selectors;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy