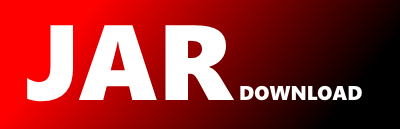
com.pulumi.kubernetes.apps.v1beta2.inputs.ReplicaSetSpecPatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.apps.v1beta2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.kubernetes.core.v1.inputs.PodTemplateSpecPatchArgs;
import com.pulumi.kubernetes.meta.v1.inputs.LabelSelectorPatchArgs;
import java.lang.Integer;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ReplicaSetSpec is the specification of a ReplicaSet.
*
*/
public final class ReplicaSetSpecPatchArgs extends com.pulumi.resources.ResourceArgs {
public static final ReplicaSetSpecPatchArgs Empty = new ReplicaSetSpecPatchArgs();
/**
* Minimum number of seconds for which a newly created pod should be ready without any of its container crashing, for it to be considered available. Defaults to 0 (pod will be considered available as soon as it is ready)
*
*/
@Import(name="minReadySeconds")
private @Nullable Output minReadySeconds;
/**
* @return Minimum number of seconds for which a newly created pod should be ready without any of its container crashing, for it to be considered available. Defaults to 0 (pod will be considered available as soon as it is ready)
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy