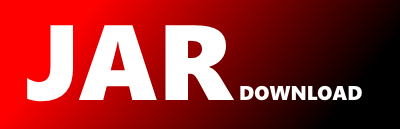
com.pulumi.kubernetes.autoscaling.v2beta1.inputs.ObjectMetricStatusArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.autoscaling.v2beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.autoscaling.v2beta1.inputs.CrossVersionObjectReferenceArgs;
import com.pulumi.kubernetes.meta.v1.inputs.LabelSelectorArgs;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ObjectMetricStatus indicates the current value of a metric describing a kubernetes object (for example, hits-per-second on an Ingress object).
*
*/
public final class ObjectMetricStatusArgs extends com.pulumi.resources.ResourceArgs {
public static final ObjectMetricStatusArgs Empty = new ObjectMetricStatusArgs();
/**
* averageValue is the current value of the average of the metric across all relevant pods (as a quantity)
*
*/
@Import(name="averageValue")
private @Nullable Output averageValue;
/**
* @return averageValue is the current value of the average of the metric across all relevant pods (as a quantity)
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy