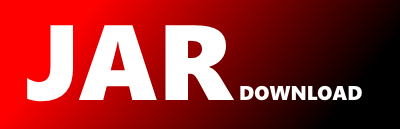
com.pulumi.kubernetes.resource.v1alpha3.inputs.ResourcePoolArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.resource.v1alpha3.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
/**
* ResourcePool describes the pool that ResourceSlices belong to.
*
*/
public final class ResourcePoolArgs extends com.pulumi.resources.ResourceArgs {
public static final ResourcePoolArgs Empty = new ResourcePoolArgs();
/**
* Generation tracks the change in a pool over time. Whenever a driver changes something about one or more of the resources in a pool, it must change the generation in all ResourceSlices which are part of that pool. Consumers of ResourceSlices should only consider resources from the pool with the highest generation number. The generation may be reset by drivers, which should be fine for consumers, assuming that all ResourceSlices in a pool are updated to match or deleted.
*
* Combined with ResourceSliceCount, this mechanism enables consumers to detect pools which are comprised of multiple ResourceSlices and are in an incomplete state.
*
*/
@Import(name="generation", required=true)
private Output generation;
/**
* @return Generation tracks the change in a pool over time. Whenever a driver changes something about one or more of the resources in a pool, it must change the generation in all ResourceSlices which are part of that pool. Consumers of ResourceSlices should only consider resources from the pool with the highest generation number. The generation may be reset by drivers, which should be fine for consumers, assuming that all ResourceSlices in a pool are updated to match or deleted.
*
* Combined with ResourceSliceCount, this mechanism enables consumers to detect pools which are comprised of multiple ResourceSlices and are in an incomplete state.
*
*/
public Output generation() {
return this.generation;
}
/**
* Name is used to identify the pool. For node-local devices, this is often the node name, but this is not required.
*
* It must not be longer than 253 characters and must consist of one or more DNS sub-domains separated by slashes. This field is immutable.
*
*/
@Import(name="name", required=true)
private Output name;
/**
* @return Name is used to identify the pool. For node-local devices, this is often the node name, but this is not required.
*
* It must not be longer than 253 characters and must consist of one or more DNS sub-domains separated by slashes. This field is immutable.
*
*/
public Output name() {
return this.name;
}
/**
* ResourceSliceCount is the total number of ResourceSlices in the pool at this generation number. Must be greater than zero.
*
* Consumers can use this to check whether they have seen all ResourceSlices belonging to the same pool.
*
*/
@Import(name="resourceSliceCount", required=true)
private Output resourceSliceCount;
/**
* @return ResourceSliceCount is the total number of ResourceSlices in the pool at this generation number. Must be greater than zero.
*
* Consumers can use this to check whether they have seen all ResourceSlices belonging to the same pool.
*
*/
public Output resourceSliceCount() {
return this.resourceSliceCount;
}
private ResourcePoolArgs() {}
private ResourcePoolArgs(ResourcePoolArgs $) {
this.generation = $.generation;
this.name = $.name;
this.resourceSliceCount = $.resourceSliceCount;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ResourcePoolArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private ResourcePoolArgs $;
public Builder() {
$ = new ResourcePoolArgs();
}
public Builder(ResourcePoolArgs defaults) {
$ = new ResourcePoolArgs(Objects.requireNonNull(defaults));
}
/**
* @param generation Generation tracks the change in a pool over time. Whenever a driver changes something about one or more of the resources in a pool, it must change the generation in all ResourceSlices which are part of that pool. Consumers of ResourceSlices should only consider resources from the pool with the highest generation number. The generation may be reset by drivers, which should be fine for consumers, assuming that all ResourceSlices in a pool are updated to match or deleted.
*
* Combined with ResourceSliceCount, this mechanism enables consumers to detect pools which are comprised of multiple ResourceSlices and are in an incomplete state.
*
* @return builder
*
*/
public Builder generation(Output generation) {
$.generation = generation;
return this;
}
/**
* @param generation Generation tracks the change in a pool over time. Whenever a driver changes something about one or more of the resources in a pool, it must change the generation in all ResourceSlices which are part of that pool. Consumers of ResourceSlices should only consider resources from the pool with the highest generation number. The generation may be reset by drivers, which should be fine for consumers, assuming that all ResourceSlices in a pool are updated to match or deleted.
*
* Combined with ResourceSliceCount, this mechanism enables consumers to detect pools which are comprised of multiple ResourceSlices and are in an incomplete state.
*
* @return builder
*
*/
public Builder generation(Integer generation) {
return generation(Output.of(generation));
}
/**
* @param name Name is used to identify the pool. For node-local devices, this is often the node name, but this is not required.
*
* It must not be longer than 253 characters and must consist of one or more DNS sub-domains separated by slashes. This field is immutable.
*
* @return builder
*
*/
public Builder name(Output name) {
$.name = name;
return this;
}
/**
* @param name Name is used to identify the pool. For node-local devices, this is often the node name, but this is not required.
*
* It must not be longer than 253 characters and must consist of one or more DNS sub-domains separated by slashes. This field is immutable.
*
* @return builder
*
*/
public Builder name(String name) {
return name(Output.of(name));
}
/**
* @param resourceSliceCount ResourceSliceCount is the total number of ResourceSlices in the pool at this generation number. Must be greater than zero.
*
* Consumers can use this to check whether they have seen all ResourceSlices belonging to the same pool.
*
* @return builder
*
*/
public Builder resourceSliceCount(Output resourceSliceCount) {
$.resourceSliceCount = resourceSliceCount;
return this;
}
/**
* @param resourceSliceCount ResourceSliceCount is the total number of ResourceSlices in the pool at this generation number. Must be greater than zero.
*
* Consumers can use this to check whether they have seen all ResourceSlices belonging to the same pool.
*
* @return builder
*
*/
public Builder resourceSliceCount(Integer resourceSliceCount) {
return resourceSliceCount(Output.of(resourceSliceCount));
}
public ResourcePoolArgs build() {
if ($.generation == null) {
throw new MissingRequiredPropertyException("ResourcePoolArgs", "generation");
}
if ($.name == null) {
throw new MissingRequiredPropertyException("ResourcePoolArgs", "name");
}
if ($.resourceSliceCount == null) {
throw new MissingRequiredPropertyException("ResourcePoolArgs", "resourceSliceCount");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy