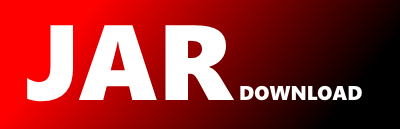
com.pulumi.kubernetes.settings.v1alpha1.outputs.PodPresetSpecPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.settings.v1alpha1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.kubernetes.core.v1.outputs.EnvFromSourcePatch;
import com.pulumi.kubernetes.core.v1.outputs.EnvVarPatch;
import com.pulumi.kubernetes.core.v1.outputs.VolumeMountPatch;
import com.pulumi.kubernetes.core.v1.outputs.VolumePatch;
import com.pulumi.kubernetes.meta.v1.outputs.LabelSelectorPatch;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class PodPresetSpecPatch {
/**
* @return Env defines the collection of EnvVar to inject into containers.
*
*/
private @Nullable List env;
/**
* @return EnvFrom defines the collection of EnvFromSource to inject into containers.
*
*/
private @Nullable List envFrom;
/**
* @return Selector is a label query over a set of resources, in this case pods. Required.
*
*/
private @Nullable LabelSelectorPatch selector;
/**
* @return VolumeMounts defines the collection of VolumeMount to inject into containers.
*
*/
private @Nullable List volumeMounts;
/**
* @return Volumes defines the collection of Volume to inject into the pod.
*
*/
private @Nullable List volumes;
private PodPresetSpecPatch() {}
/**
* @return Env defines the collection of EnvVar to inject into containers.
*
*/
public List env() {
return this.env == null ? List.of() : this.env;
}
/**
* @return EnvFrom defines the collection of EnvFromSource to inject into containers.
*
*/
public List envFrom() {
return this.envFrom == null ? List.of() : this.envFrom;
}
/**
* @return Selector is a label query over a set of resources, in this case pods. Required.
*
*/
public Optional selector() {
return Optional.ofNullable(this.selector);
}
/**
* @return VolumeMounts defines the collection of VolumeMount to inject into containers.
*
*/
public List volumeMounts() {
return this.volumeMounts == null ? List.of() : this.volumeMounts;
}
/**
* @return Volumes defines the collection of Volume to inject into the pod.
*
*/
public List volumes() {
return this.volumes == null ? List.of() : this.volumes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PodPresetSpecPatch defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List env;
private @Nullable List envFrom;
private @Nullable LabelSelectorPatch selector;
private @Nullable List volumeMounts;
private @Nullable List volumes;
public Builder() {}
public Builder(PodPresetSpecPatch defaults) {
Objects.requireNonNull(defaults);
this.env = defaults.env;
this.envFrom = defaults.envFrom;
this.selector = defaults.selector;
this.volumeMounts = defaults.volumeMounts;
this.volumes = defaults.volumes;
}
@CustomType.Setter
public Builder env(@Nullable List env) {
this.env = env;
return this;
}
public Builder env(EnvVarPatch... env) {
return env(List.of(env));
}
@CustomType.Setter
public Builder envFrom(@Nullable List envFrom) {
this.envFrom = envFrom;
return this;
}
public Builder envFrom(EnvFromSourcePatch... envFrom) {
return envFrom(List.of(envFrom));
}
@CustomType.Setter
public Builder selector(@Nullable LabelSelectorPatch selector) {
this.selector = selector;
return this;
}
@CustomType.Setter
public Builder volumeMounts(@Nullable List volumeMounts) {
this.volumeMounts = volumeMounts;
return this;
}
public Builder volumeMounts(VolumeMountPatch... volumeMounts) {
return volumeMounts(List.of(volumeMounts));
}
@CustomType.Setter
public Builder volumes(@Nullable List volumes) {
this.volumes = volumes;
return this;
}
public Builder volumes(VolumePatch... volumes) {
return volumes(List.of(volumes));
}
public PodPresetSpecPatch build() {
final var _resultValue = new PodPresetSpecPatch();
_resultValue.env = env;
_resultValue.envFrom = envFrom;
_resultValue.selector = selector;
_resultValue.volumeMounts = volumeMounts;
_resultValue.volumes = volumes;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy