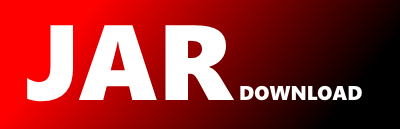
com.pulumi.kubernetes.core.v1.SecretPatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.core.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.kubernetes.meta.v1.inputs.ObjectMetaPatchArgs;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SecretPatchArgs extends com.pulumi.resources.ResourceArgs {
public static final SecretPatchArgs Empty = new SecretPatchArgs();
/**
* APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*
*/
@Import(name="apiVersion")
private @Nullable Output apiVersion;
/**
* @return APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy