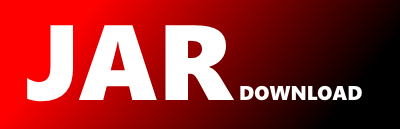
com.pulumi.kubernetes.apps.v1.inputs.DaemonSetStatusArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.apps.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.apps.v1.inputs.DaemonSetConditionArgs;
import java.lang.Integer;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* DaemonSetStatus represents the current status of a daemon set.
*
*/
public final class DaemonSetStatusArgs extends com.pulumi.resources.ResourceArgs {
public static final DaemonSetStatusArgs Empty = new DaemonSetStatusArgs();
/**
* Count of hash collisions for the DaemonSet. The DaemonSet controller uses this field as a collision avoidance mechanism when it needs to create the name for the newest ControllerRevision.
*
*/
@Import(name="collisionCount")
private @Nullable Output collisionCount;
/**
* @return Count of hash collisions for the DaemonSet. The DaemonSet controller uses this field as a collision avoidance mechanism when it needs to create the name for the newest ControllerRevision.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy