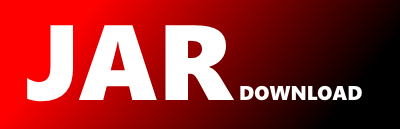
com.pulumi.kubernetes.apiextensions.v1.inputs.CustomResourceColumnDefinitionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.apiextensions.v1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* CustomResourceColumnDefinition specifies a column for server side printing.
*
*/
public final class CustomResourceColumnDefinitionArgs extends com.pulumi.resources.ResourceArgs {
public static final CustomResourceColumnDefinitionArgs Empty = new CustomResourceColumnDefinitionArgs();
/**
* description is a human readable description of this column.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return description is a human readable description of this column.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy