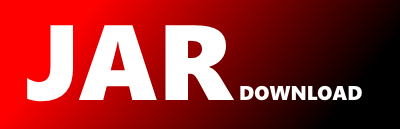
com.pulumi.kubernetes.autoscaling.v2.outputs.HorizontalPodAutoscalerStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.autoscaling.v2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.autoscaling.v2.outputs.HorizontalPodAutoscalerCondition;
import com.pulumi.kubernetes.autoscaling.v2.outputs.MetricStatus;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class HorizontalPodAutoscalerStatus {
/**
* @return conditions is the set of conditions required for this autoscaler to scale its target, and indicates whether or not those conditions are met.
*
*/
private @Nullable List conditions;
/**
* @return currentMetrics is the last read state of the metrics used by this autoscaler.
*
*/
private @Nullable List currentMetrics;
/**
* @return currentReplicas is current number of replicas of pods managed by this autoscaler, as last seen by the autoscaler.
*
*/
private @Nullable Integer currentReplicas;
/**
* @return desiredReplicas is the desired number of replicas of pods managed by this autoscaler, as last calculated by the autoscaler.
*
*/
private Integer desiredReplicas;
/**
* @return lastScaleTime is the last time the HorizontalPodAutoscaler scaled the number of pods, used by the autoscaler to control how often the number of pods is changed.
*
*/
private @Nullable String lastScaleTime;
/**
* @return observedGeneration is the most recent generation observed by this autoscaler.
*
*/
private @Nullable Integer observedGeneration;
private HorizontalPodAutoscalerStatus() {}
/**
* @return conditions is the set of conditions required for this autoscaler to scale its target, and indicates whether or not those conditions are met.
*
*/
public List conditions() {
return this.conditions == null ? List.of() : this.conditions;
}
/**
* @return currentMetrics is the last read state of the metrics used by this autoscaler.
*
*/
public List currentMetrics() {
return this.currentMetrics == null ? List.of() : this.currentMetrics;
}
/**
* @return currentReplicas is current number of replicas of pods managed by this autoscaler, as last seen by the autoscaler.
*
*/
public Optional currentReplicas() {
return Optional.ofNullable(this.currentReplicas);
}
/**
* @return desiredReplicas is the desired number of replicas of pods managed by this autoscaler, as last calculated by the autoscaler.
*
*/
public Integer desiredReplicas() {
return this.desiredReplicas;
}
/**
* @return lastScaleTime is the last time the HorizontalPodAutoscaler scaled the number of pods, used by the autoscaler to control how often the number of pods is changed.
*
*/
public Optional lastScaleTime() {
return Optional.ofNullable(this.lastScaleTime);
}
/**
* @return observedGeneration is the most recent generation observed by this autoscaler.
*
*/
public Optional observedGeneration() {
return Optional.ofNullable(this.observedGeneration);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HorizontalPodAutoscalerStatus defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List conditions;
private @Nullable List currentMetrics;
private @Nullable Integer currentReplicas;
private Integer desiredReplicas;
private @Nullable String lastScaleTime;
private @Nullable Integer observedGeneration;
public Builder() {}
public Builder(HorizontalPodAutoscalerStatus defaults) {
Objects.requireNonNull(defaults);
this.conditions = defaults.conditions;
this.currentMetrics = defaults.currentMetrics;
this.currentReplicas = defaults.currentReplicas;
this.desiredReplicas = defaults.desiredReplicas;
this.lastScaleTime = defaults.lastScaleTime;
this.observedGeneration = defaults.observedGeneration;
}
@CustomType.Setter
public Builder conditions(@Nullable List conditions) {
this.conditions = conditions;
return this;
}
public Builder conditions(HorizontalPodAutoscalerCondition... conditions) {
return conditions(List.of(conditions));
}
@CustomType.Setter
public Builder currentMetrics(@Nullable List currentMetrics) {
this.currentMetrics = currentMetrics;
return this;
}
public Builder currentMetrics(MetricStatus... currentMetrics) {
return currentMetrics(List.of(currentMetrics));
}
@CustomType.Setter
public Builder currentReplicas(@Nullable Integer currentReplicas) {
this.currentReplicas = currentReplicas;
return this;
}
@CustomType.Setter
public Builder desiredReplicas(Integer desiredReplicas) {
if (desiredReplicas == null) {
throw new MissingRequiredPropertyException("HorizontalPodAutoscalerStatus", "desiredReplicas");
}
this.desiredReplicas = desiredReplicas;
return this;
}
@CustomType.Setter
public Builder lastScaleTime(@Nullable String lastScaleTime) {
this.lastScaleTime = lastScaleTime;
return this;
}
@CustomType.Setter
public Builder observedGeneration(@Nullable Integer observedGeneration) {
this.observedGeneration = observedGeneration;
return this;
}
public HorizontalPodAutoscalerStatus build() {
final var _resultValue = new HorizontalPodAutoscalerStatus();
_resultValue.conditions = conditions;
_resultValue.currentMetrics = currentMetrics;
_resultValue.currentReplicas = currentReplicas;
_resultValue.desiredReplicas = desiredReplicas;
_resultValue.lastScaleTime = lastScaleTime;
_resultValue.observedGeneration = observedGeneration;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy