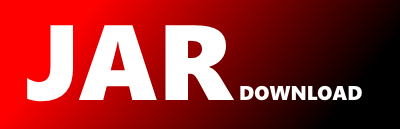
com.pulumi.kubernetes.extensions.v1beta1.outputs.HTTPIngressPath Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.extensions.v1beta1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.IngressBackend;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class HTTPIngressPath {
/**
* @return Backend defines the referenced service endpoint to which the traffic will be forwarded to.
*
*/
private IngressBackend backend;
/**
* @return Path is an extended POSIX regex as defined by IEEE Std 1003.1, (i.e this follows the egrep/unix syntax, not the perl syntax) matched against the path of an incoming request. Currently it can contain characters disallowed from the conventional "path" part of a URL as defined by RFC 3986. Paths must begin with a '/'. If unspecified, the path defaults to a catch all sending traffic to the backend.
*
*/
private @Nullable String path;
/**
* @return PathType determines the interpretation of the Path matching. PathType can be one of the following values: * Exact: Matches the URL path exactly. * Prefix: Matches based on a URL path prefix split by '/'. Matching is
* done on a path element by element basis. A path element refers is the
* list of labels in the path split by the '/' separator. A request is a
* match for path p if every p is an element-wise prefix of p of the
* request path. Note that if the last element of the path is a substring
* of the last element in request path, it is not a match (e.g. /foo/bar
* matches /foo/bar/baz, but does not match /foo/barbaz).
* * ImplementationSpecific: Interpretation of the Path matching is up to
* the IngressClass. Implementations can treat this as a separate PathType
* or treat it identically to Prefix or Exact path types.
* Implementations are required to support all path types. Defaults to ImplementationSpecific.
*
*/
private @Nullable String pathType;
private HTTPIngressPath() {}
/**
* @return Backend defines the referenced service endpoint to which the traffic will be forwarded to.
*
*/
public IngressBackend backend() {
return this.backend;
}
/**
* @return Path is an extended POSIX regex as defined by IEEE Std 1003.1, (i.e this follows the egrep/unix syntax, not the perl syntax) matched against the path of an incoming request. Currently it can contain characters disallowed from the conventional "path" part of a URL as defined by RFC 3986. Paths must begin with a '/'. If unspecified, the path defaults to a catch all sending traffic to the backend.
*
*/
public Optional path() {
return Optional.ofNullable(this.path);
}
/**
* @return PathType determines the interpretation of the Path matching. PathType can be one of the following values: * Exact: Matches the URL path exactly. * Prefix: Matches based on a URL path prefix split by '/'. Matching is
* done on a path element by element basis. A path element refers is the
* list of labels in the path split by the '/' separator. A request is a
* match for path p if every p is an element-wise prefix of p of the
* request path. Note that if the last element of the path is a substring
* of the last element in request path, it is not a match (e.g. /foo/bar
* matches /foo/bar/baz, but does not match /foo/barbaz).
* * ImplementationSpecific: Interpretation of the Path matching is up to
* the IngressClass. Implementations can treat this as a separate PathType
* or treat it identically to Prefix or Exact path types.
* Implementations are required to support all path types. Defaults to ImplementationSpecific.
*
*/
public Optional pathType() {
return Optional.ofNullable(this.pathType);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(HTTPIngressPath defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private IngressBackend backend;
private @Nullable String path;
private @Nullable String pathType;
public Builder() {}
public Builder(HTTPIngressPath defaults) {
Objects.requireNonNull(defaults);
this.backend = defaults.backend;
this.path = defaults.path;
this.pathType = defaults.pathType;
}
@CustomType.Setter
public Builder backend(IngressBackend backend) {
if (backend == null) {
throw new MissingRequiredPropertyException("HTTPIngressPath", "backend");
}
this.backend = backend;
return this;
}
@CustomType.Setter
public Builder path(@Nullable String path) {
this.path = path;
return this;
}
@CustomType.Setter
public Builder pathType(@Nullable String pathType) {
this.pathType = pathType;
return this;
}
public HTTPIngressPath build() {
final var _resultValue = new HTTPIngressPath();
_resultValue.backend = backend;
_resultValue.path = path;
_resultValue.pathType = pathType;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy