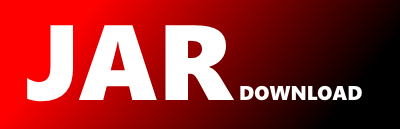
com.pulumi.kubernetes.helm.v3.Release Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.helm.v3;
import com.pulumi.asset.AssetOrArchive;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.kubernetes.Utilities;
import com.pulumi.kubernetes.helm.v3.ReleaseArgs;
import com.pulumi.kubernetes.helm.v3.outputs.ReleaseStatus;
import com.pulumi.kubernetes.helm.v3.outputs.RepositoryOpts;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* A `Release` is an instance of a chart running in a Kubernetes cluster. A `Chart` is a Helm package. It contains all the
* resource definitions necessary to run an application, tool, or service inside a Kubernetes cluster.
*
* This resource models a Helm Release as if it were created by the Helm CLI. The underlying implementation embeds Helm as
* a library to perform the orchestration of the resources. As a result, the full spectrum of Helm features are supported
* natively.
*
* You may also want to consider the `Chart` resource as an alternative method for managing helm charts. For more information about the trade-offs between these options see: [Choosing the right Helm resource for your use case](https://www.pulumi.com/registry/packages/kubernetes/how-to-guides/choosing-the-right-helm-resource-for-your-use-case)
*
* ## Example Usage
*
* ## Import
*
* An existing Helm Release resource can be imported using its `type token`, `name` and identifier, e.g.
*
* ```sh
* $ pulumi import kubernetes:helm.sh/v3:Release myRelease <namespace>/<releaseName>
* ```
*
*/
@ResourceType(type="kubernetes:helm.sh/v3:Release")
public class Release extends com.pulumi.resources.CustomResource {
/**
* Whether to allow Null values in helm chart configs.
*
*/
@Export(name="allowNullValues", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> allowNullValues;
/**
* @return Whether to allow Null values in helm chart configs.
*
*/
public Output> allowNullValues() {
return Codegen.optional(this.allowNullValues);
}
/**
* If set, installation process purges chart on fail. `skipAwait` will be disabled automatically if atomic is used.
*
*/
@Export(name="atomic", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> atomic;
/**
* @return If set, installation process purges chart on fail. `skipAwait` will be disabled automatically if atomic is used.
*
*/
public Output> atomic() {
return Codegen.optional(this.atomic);
}
/**
* Chart name to be installed. A path may be used.
*
*/
@Export(name="chart", refs={String.class}, tree="[0]")
private Output chart;
/**
* @return Chart name to be installed. A path may be used.
*
*/
public Output chart() {
return this.chart;
}
/**
* Allow deletion of new resources created in this upgrade when upgrade fails.
*
*/
@Export(name="cleanupOnFail", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> cleanupOnFail;
/**
* @return Allow deletion of new resources created in this upgrade when upgrade fails.
*
*/
public Output> cleanupOnFail() {
return Codegen.optional(this.cleanupOnFail);
}
/**
* Create the namespace if it does not exist.
*
*/
@Export(name="createNamespace", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> createNamespace;
/**
* @return Create the namespace if it does not exist.
*
*/
public Output> createNamespace() {
return Codegen.optional(this.createNamespace);
}
/**
* Run helm dependency update before installing the chart.
*
*/
@Export(name="dependencyUpdate", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> dependencyUpdate;
/**
* @return Run helm dependency update before installing the chart.
*
*/
public Output> dependencyUpdate() {
return Codegen.optional(this.dependencyUpdate);
}
/**
* Add a custom description
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Add a custom description
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Use chart development versions, too. Equivalent to version '>0.0.0-0'. If `version` is set, this is ignored.
*
*/
@Export(name="devel", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> devel;
/**
* @return Use chart development versions, too. Equivalent to version '>0.0.0-0'. If `version` is set, this is ignored.
*
*/
public Output> devel() {
return Codegen.optional(this.devel);
}
/**
* Prevent CRD hooks from running, but run other hooks. See helm install --no-crd-hook
*
*/
@Export(name="disableCRDHooks", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disableCRDHooks;
/**
* @return Prevent CRD hooks from running, but run other hooks. See helm install --no-crd-hook
*
*/
public Output> disableCRDHooks() {
return Codegen.optional(this.disableCRDHooks);
}
/**
* If set, the installation process will not validate rendered templates against the Kubernetes OpenAPI Schema
*
*/
@Export(name="disableOpenapiValidation", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disableOpenapiValidation;
/**
* @return If set, the installation process will not validate rendered templates against the Kubernetes OpenAPI Schema
*
*/
public Output> disableOpenapiValidation() {
return Codegen.optional(this.disableOpenapiValidation);
}
/**
* Prevent hooks from running.
*
*/
@Export(name="disableWebhooks", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disableWebhooks;
/**
* @return Prevent hooks from running.
*
*/
public Output> disableWebhooks() {
return Codegen.optional(this.disableWebhooks);
}
/**
* Force resource update through delete/recreate if needed.
*
*/
@Export(name="forceUpdate", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> forceUpdate;
/**
* @return Force resource update through delete/recreate if needed.
*
*/
public Output> forceUpdate() {
return Codegen.optional(this.forceUpdate);
}
/**
* Location of public keys used for verification. Used only if `verify` is true
*
*/
@Export(name="keyring", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> keyring;
/**
* @return Location of public keys used for verification. Used only if `verify` is true
*
*/
public Output> keyring() {
return Codegen.optional(this.keyring);
}
/**
* Run helm lint when planning.
*
*/
@Export(name="lint", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> lint;
/**
* @return Run helm lint when planning.
*
*/
public Output> lint() {
return Codegen.optional(this.lint);
}
/**
* The rendered manifests as JSON. Not yet supported.
*
*/
@Export(name="manifest", refs={Map.class,String.class,Object.class}, tree="[0,1,2]")
private Output* @Nullable */ Map> manifest;
/**
* @return The rendered manifests as JSON. Not yet supported.
*
*/
public Output>> manifest() {
return Codegen.optional(this.manifest);
}
/**
* Limit the maximum number of revisions saved per release. Use 0 for no limit.
*
*/
@Export(name="maxHistory", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> maxHistory;
/**
* @return Limit the maximum number of revisions saved per release. Use 0 for no limit.
*
*/
public Output> maxHistory() {
return Codegen.optional(this.maxHistory);
}
/**
* Release name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> name;
/**
* @return Release name.
*
*/
public Output> name() {
return Codegen.optional(this.name);
}
/**
* Namespace to install the release into.
*
*/
@Export(name="namespace", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> namespace;
/**
* @return Namespace to install the release into.
*
*/
public Output> namespace() {
return Codegen.optional(this.namespace);
}
/**
* Postrender command to run.
*
*/
@Export(name="postrender", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> postrender;
/**
* @return Postrender command to run.
*
*/
public Output> postrender() {
return Codegen.optional(this.postrender);
}
/**
* Perform pods restart during upgrade/rollback.
*
*/
@Export(name="recreatePods", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> recreatePods;
/**
* @return Perform pods restart during upgrade/rollback.
*
*/
public Output> recreatePods() {
return Codegen.optional(this.recreatePods);
}
/**
* If set, render subchart notes along with the parent.
*
*/
@Export(name="renderSubchartNotes", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> renderSubchartNotes;
/**
* @return If set, render subchart notes along with the parent.
*
*/
public Output> renderSubchartNotes() {
return Codegen.optional(this.renderSubchartNotes);
}
/**
* Re-use the given name, even if that name is already used. This is unsafe in production
*
*/
@Export(name="replace", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> replace;
/**
* @return Re-use the given name, even if that name is already used. This is unsafe in production
*
*/
public Output> replace() {
return Codegen.optional(this.replace);
}
/**
* Specification defining the Helm chart repository to use.
*
*/
@Export(name="repositoryOpts", refs={RepositoryOpts.class}, tree="[0]")
private Output* @Nullable */ RepositoryOpts> repositoryOpts;
/**
* @return Specification defining the Helm chart repository to use.
*
*/
public Output> repositoryOpts() {
return Codegen.optional(this.repositoryOpts);
}
/**
* When upgrading, reset the values to the ones built into the chart.
*
*/
@Export(name="resetValues", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> resetValues;
/**
* @return When upgrading, reset the values to the ones built into the chart.
*
*/
public Output> resetValues() {
return Codegen.optional(this.resetValues);
}
/**
* Names of resources created by the release grouped by "kind/version".
*
*/
@Export(name="resourceNames", refs={Map.class,String.class,List.class}, tree="[0,1,[2,1]]")
private Output* @Nullable */ Map>> resourceNames;
/**
* @return Names of resources created by the release grouped by "kind/version".
*
*/
public Output>>> resourceNames() {
return Codegen.optional(this.resourceNames);
}
/**
* When upgrading, reuse the last release's values and merge in any overrides. If 'resetValues' is specified, this is ignored
*
*/
@Export(name="reuseValues", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> reuseValues;
/**
* @return When upgrading, reuse the last release's values and merge in any overrides. If 'resetValues' is specified, this is ignored
*
*/
public Output> reuseValues() {
return Codegen.optional(this.reuseValues);
}
/**
* By default, the provider waits until all resources are in a ready state before marking the release as successful. Setting this to true will skip such await logic.
*
*/
@Export(name="skipAwait", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> skipAwait;
/**
* @return By default, the provider waits until all resources are in a ready state before marking the release as successful. Setting this to true will skip such await logic.
*
*/
public Output> skipAwait() {
return Codegen.optional(this.skipAwait);
}
/**
* If set, no CRDs will be installed. By default, CRDs are installed if not already present.
*
*/
@Export(name="skipCrds", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> skipCrds;
/**
* @return If set, no CRDs will be installed. By default, CRDs are installed if not already present.
*
*/
public Output> skipCrds() {
return Codegen.optional(this.skipCrds);
}
/**
* Status of the deployed release.
*
*/
@Export(name="status", refs={ReleaseStatus.class}, tree="[0]")
private Output status;
/**
* @return Status of the deployed release.
*
*/
public Output status() {
return this.status;
}
/**
* Time in seconds to wait for any individual kubernetes operation.
*
*/
@Export(name="timeout", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> timeout;
/**
* @return Time in seconds to wait for any individual kubernetes operation.
*
*/
public Output> timeout() {
return Codegen.optional(this.timeout);
}
/**
* List of assets (raw yaml files). Content is read and merged with values (with values taking precedence).
*
*/
@Export(name="valueYamlFiles", refs={List.class,AssetOrArchive.class}, tree="[0,1]")
private Output* @Nullable */ List> valueYamlFiles;
/**
* @return List of assets (raw yaml files). Content is read and merged with values (with values taking precedence).
*
*/
public Output>> valueYamlFiles() {
return Codegen.optional(this.valueYamlFiles);
}
/**
* Custom values set for the release.
*
*/
@Export(name="values", refs={Map.class,String.class,Object.class}, tree="[0,1,2]")
private Output* @Nullable */ Map> values;
/**
* @return Custom values set for the release.
*
*/
public Output>> values() {
return Codegen.optional(this.values);
}
/**
* Verify the package before installing it.
*
*/
@Export(name="verify", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> verify;
/**
* @return Verify the package before installing it.
*
*/
public Output> verify() {
return Codegen.optional(this.verify);
}
/**
* Specify the exact chart version to install. If this is not specified, the latest version is installed.
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> version;
/**
* @return Specify the exact chart version to install. If this is not specified, the latest version is installed.
*
*/
public Output> version() {
return Codegen.optional(this.version);
}
/**
* Will wait until all Jobs have been completed before marking the release as successful. This is ignored if `skipAwait` is enabled.
*
*/
@Export(name="waitForJobs", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> waitForJobs;
/**
* @return Will wait until all Jobs have been completed before marking the release as successful. This is ignored if `skipAwait` is enabled.
*
*/
public Output> waitForJobs() {
return Codegen.optional(this.waitForJobs);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Release(String name) {
this(name, ReleaseArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Release(String name, ReleaseArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Release(String name, ReleaseArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("kubernetes:helm.sh/v3:Release", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()));
}
private Release(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("kubernetes:helm.sh/v3:Release", name, null, makeResourceOptions(options, id));
}
private static ReleaseArgs makeArgs(ReleaseArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
var builder = args == null ? ReleaseArgs.builder() : ReleaseArgs.builder(args);
return builder
.compat("true")
.build();
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Release get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Release(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy