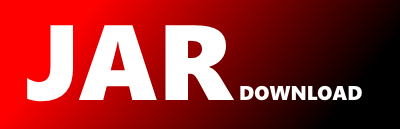
com.pulumi.kubernetes.autoscaling.v2beta1.inputs.HorizontalPodAutoscalerStatusArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.autoscaling.v2beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.autoscaling.v2beta1.inputs.HorizontalPodAutoscalerConditionArgs;
import com.pulumi.kubernetes.autoscaling.v2beta1.inputs.MetricStatusArgs;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* HorizontalPodAutoscalerStatus describes the current status of a horizontal pod autoscaler.
*
*/
public final class HorizontalPodAutoscalerStatusArgs extends com.pulumi.resources.ResourceArgs {
public static final HorizontalPodAutoscalerStatusArgs Empty = new HorizontalPodAutoscalerStatusArgs();
/**
* conditions is the set of conditions required for this autoscaler to scale its target, and indicates whether or not those conditions are met.
*
*/
@Import(name="conditions", required=true)
private Output> conditions;
/**
* @return conditions is the set of conditions required for this autoscaler to scale its target, and indicates whether or not those conditions are met.
*
*/
public Output> conditions() {
return this.conditions;
}
/**
* currentMetrics is the last read state of the metrics used by this autoscaler.
*
*/
@Import(name="currentMetrics")
private @Nullable Output> currentMetrics;
/**
* @return currentMetrics is the last read state of the metrics used by this autoscaler.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy