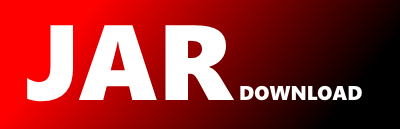
com.pulumi.kubernetes.meta.v1.inputs.ManagedFieldsEntryArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.meta.v1.inputs;
import com.google.gson.JsonElement;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ManagedFieldsEntry is a workflow-id, a FieldSet and the group version of the resource that the fieldset applies to.
*
*/
public final class ManagedFieldsEntryArgs extends com.pulumi.resources.ResourceArgs {
public static final ManagedFieldsEntryArgs Empty = new ManagedFieldsEntryArgs();
/**
* APIVersion defines the version of this resource that this field set applies to. The format is "group/version" just like the top-level APIVersion field. It is necessary to track the version of a field set because it cannot be automatically converted.
*
*/
@Import(name="apiVersion")
private @Nullable Output apiVersion;
/**
* @return APIVersion defines the version of this resource that this field set applies to. The format is "group/version" just like the top-level APIVersion field. It is necessary to track the version of a field set because it cannot be automatically converted.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy