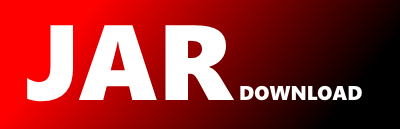
com.pulumi.kubernetes.apps.v1.outputs.DaemonSetStatusPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.apps.v1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.kubernetes.apps.v1.outputs.DaemonSetConditionPatch;
import java.lang.Integer;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class DaemonSetStatusPatch {
/**
* @return Count of hash collisions for the DaemonSet. The DaemonSet controller uses this field as a collision avoidance mechanism when it needs to create the name for the newest ControllerRevision.
*
*/
private @Nullable Integer collisionCount;
/**
* @return Represents the latest available observations of a DaemonSet's current state.
*
*/
private @Nullable List conditions;
/**
* @return The number of nodes that are running at least 1 daemon pod and are supposed to run the daemon pod. More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
*
*/
private @Nullable Integer currentNumberScheduled;
/**
* @return The total number of nodes that should be running the daemon pod (including nodes correctly running the daemon pod). More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
*
*/
private @Nullable Integer desiredNumberScheduled;
/**
* @return The number of nodes that should be running the daemon pod and have one or more of the daemon pod running and available (ready for at least spec.minReadySeconds)
*
*/
private @Nullable Integer numberAvailable;
/**
* @return The number of nodes that are running the daemon pod, but are not supposed to run the daemon pod. More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
*
*/
private @Nullable Integer numberMisscheduled;
/**
* @return numberReady is the number of nodes that should be running the daemon pod and have one or more of the daemon pod running with a Ready Condition.
*
*/
private @Nullable Integer numberReady;
/**
* @return The number of nodes that should be running the daemon pod and have none of the daemon pod running and available (ready for at least spec.minReadySeconds)
*
*/
private @Nullable Integer numberUnavailable;
/**
* @return The most recent generation observed by the daemon set controller.
*
*/
private @Nullable Integer observedGeneration;
/**
* @return The total number of nodes that are running updated daemon pod
*
*/
private @Nullable Integer updatedNumberScheduled;
private DaemonSetStatusPatch() {}
/**
* @return Count of hash collisions for the DaemonSet. The DaemonSet controller uses this field as a collision avoidance mechanism when it needs to create the name for the newest ControllerRevision.
*
*/
public Optional collisionCount() {
return Optional.ofNullable(this.collisionCount);
}
/**
* @return Represents the latest available observations of a DaemonSet's current state.
*
*/
public List conditions() {
return this.conditions == null ? List.of() : this.conditions;
}
/**
* @return The number of nodes that are running at least 1 daemon pod and are supposed to run the daemon pod. More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
*
*/
public Optional currentNumberScheduled() {
return Optional.ofNullable(this.currentNumberScheduled);
}
/**
* @return The total number of nodes that should be running the daemon pod (including nodes correctly running the daemon pod). More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
*
*/
public Optional desiredNumberScheduled() {
return Optional.ofNullable(this.desiredNumberScheduled);
}
/**
* @return The number of nodes that should be running the daemon pod and have one or more of the daemon pod running and available (ready for at least spec.minReadySeconds)
*
*/
public Optional numberAvailable() {
return Optional.ofNullable(this.numberAvailable);
}
/**
* @return The number of nodes that are running the daemon pod, but are not supposed to run the daemon pod. More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
*
*/
public Optional numberMisscheduled() {
return Optional.ofNullable(this.numberMisscheduled);
}
/**
* @return numberReady is the number of nodes that should be running the daemon pod and have one or more of the daemon pod running with a Ready Condition.
*
*/
public Optional numberReady() {
return Optional.ofNullable(this.numberReady);
}
/**
* @return The number of nodes that should be running the daemon pod and have none of the daemon pod running and available (ready for at least spec.minReadySeconds)
*
*/
public Optional numberUnavailable() {
return Optional.ofNullable(this.numberUnavailable);
}
/**
* @return The most recent generation observed by the daemon set controller.
*
*/
public Optional observedGeneration() {
return Optional.ofNullable(this.observedGeneration);
}
/**
* @return The total number of nodes that are running updated daemon pod
*
*/
public Optional updatedNumberScheduled() {
return Optional.ofNullable(this.updatedNumberScheduled);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(DaemonSetStatusPatch defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer collisionCount;
private @Nullable List conditions;
private @Nullable Integer currentNumberScheduled;
private @Nullable Integer desiredNumberScheduled;
private @Nullable Integer numberAvailable;
private @Nullable Integer numberMisscheduled;
private @Nullable Integer numberReady;
private @Nullable Integer numberUnavailable;
private @Nullable Integer observedGeneration;
private @Nullable Integer updatedNumberScheduled;
public Builder() {}
public Builder(DaemonSetStatusPatch defaults) {
Objects.requireNonNull(defaults);
this.collisionCount = defaults.collisionCount;
this.conditions = defaults.conditions;
this.currentNumberScheduled = defaults.currentNumberScheduled;
this.desiredNumberScheduled = defaults.desiredNumberScheduled;
this.numberAvailable = defaults.numberAvailable;
this.numberMisscheduled = defaults.numberMisscheduled;
this.numberReady = defaults.numberReady;
this.numberUnavailable = defaults.numberUnavailable;
this.observedGeneration = defaults.observedGeneration;
this.updatedNumberScheduled = defaults.updatedNumberScheduled;
}
@CustomType.Setter
public Builder collisionCount(@Nullable Integer collisionCount) {
this.collisionCount = collisionCount;
return this;
}
@CustomType.Setter
public Builder conditions(@Nullable List conditions) {
this.conditions = conditions;
return this;
}
public Builder conditions(DaemonSetConditionPatch... conditions) {
return conditions(List.of(conditions));
}
@CustomType.Setter
public Builder currentNumberScheduled(@Nullable Integer currentNumberScheduled) {
this.currentNumberScheduled = currentNumberScheduled;
return this;
}
@CustomType.Setter
public Builder desiredNumberScheduled(@Nullable Integer desiredNumberScheduled) {
this.desiredNumberScheduled = desiredNumberScheduled;
return this;
}
@CustomType.Setter
public Builder numberAvailable(@Nullable Integer numberAvailable) {
this.numberAvailable = numberAvailable;
return this;
}
@CustomType.Setter
public Builder numberMisscheduled(@Nullable Integer numberMisscheduled) {
this.numberMisscheduled = numberMisscheduled;
return this;
}
@CustomType.Setter
public Builder numberReady(@Nullable Integer numberReady) {
this.numberReady = numberReady;
return this;
}
@CustomType.Setter
public Builder numberUnavailable(@Nullable Integer numberUnavailable) {
this.numberUnavailable = numberUnavailable;
return this;
}
@CustomType.Setter
public Builder observedGeneration(@Nullable Integer observedGeneration) {
this.observedGeneration = observedGeneration;
return this;
}
@CustomType.Setter
public Builder updatedNumberScheduled(@Nullable Integer updatedNumberScheduled) {
this.updatedNumberScheduled = updatedNumberScheduled;
return this;
}
public DaemonSetStatusPatch build() {
final var _resultValue = new DaemonSetStatusPatch();
_resultValue.collisionCount = collisionCount;
_resultValue.conditions = conditions;
_resultValue.currentNumberScheduled = currentNumberScheduled;
_resultValue.desiredNumberScheduled = desiredNumberScheduled;
_resultValue.numberAvailable = numberAvailable;
_resultValue.numberMisscheduled = numberMisscheduled;
_resultValue.numberReady = numberReady;
_resultValue.numberUnavailable = numberUnavailable;
_resultValue.observedGeneration = observedGeneration;
_resultValue.updatedNumberScheduled = updatedNumberScheduled;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy