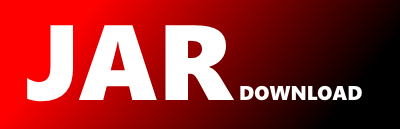
com.pulumi.kubernetes.autoscaling.v2beta1.inputs.HorizontalPodAutoscalerSpecPatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.autoscaling.v2beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.kubernetes.autoscaling.v2beta1.inputs.CrossVersionObjectReferencePatchArgs;
import com.pulumi.kubernetes.autoscaling.v2beta1.inputs.MetricSpecPatchArgs;
import java.lang.Integer;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* HorizontalPodAutoscalerSpec describes the desired functionality of the HorizontalPodAutoscaler.
*
*/
public final class HorizontalPodAutoscalerSpecPatchArgs extends com.pulumi.resources.ResourceArgs {
public static final HorizontalPodAutoscalerSpecPatchArgs Empty = new HorizontalPodAutoscalerSpecPatchArgs();
/**
* maxReplicas is the upper limit for the number of replicas to which the autoscaler can scale up. It cannot be less that minReplicas.
*
*/
@Import(name="maxReplicas")
private @Nullable Output maxReplicas;
/**
* @return maxReplicas is the upper limit for the number of replicas to which the autoscaler can scale up. It cannot be less that minReplicas.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy