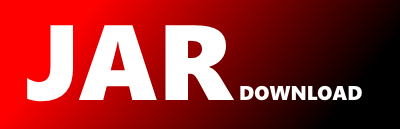
com.pulumi.kubernetes.core.v1.ConfigMapPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.core.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.kubernetes.Utilities;
import com.pulumi.kubernetes.core.v1.ConfigMapPatchArgs;
import com.pulumi.kubernetes.meta.v1.outputs.ObjectMetaPatch;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Patch resources are used to modify existing Kubernetes resources by using
* Server-Side Apply updates. The name of the resource must be specified, but all other properties are optional. More than
* one patch may be applied to the same resource, and a random FieldManager name will be used for each Patch resource.
* Conflicts will result in an error by default, but can be forced using the "pulumi.com/patchForce" annotation. See the
* [Server-Side Apply Docs](https://www.pulumi.com/registry/packages/kubernetes/how-to-guides/managing-resources-with-server-side-apply/) for
* additional information about using Server-Side Apply to manage Kubernetes resources with Pulumi.
* ConfigMap holds configuration data for pods to consume.
*
*/
@ResourceType(type="kubernetes:core/v1:ConfigMapPatch")
public class ConfigMapPatch extends com.pulumi.resources.CustomResource {
/**
* APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*
*/
@Export(name="apiVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> apiVersion;
/**
* @return APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*
*/
public Output> apiVersion() {
return Codegen.optional(this.apiVersion);
}
/**
* BinaryData contains the binary data. Each key must consist of alphanumeric characters, '-', '_' or '.'. BinaryData can contain byte sequences that are not in the UTF-8 range. The keys stored in BinaryData must not overlap with the ones in the Data field, this is enforced during validation process. Using this field will require 1.10+ apiserver and kubelet.
*
*/
@Export(name="binaryData", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> binaryData;
/**
* @return BinaryData contains the binary data. Each key must consist of alphanumeric characters, '-', '_' or '.'. BinaryData can contain byte sequences that are not in the UTF-8 range. The keys stored in BinaryData must not overlap with the ones in the Data field, this is enforced during validation process. Using this field will require 1.10+ apiserver and kubelet.
*
*/
public Output>> binaryData() {
return Codegen.optional(this.binaryData);
}
/**
* Data contains the configuration data. Each key must consist of alphanumeric characters, '-', '_' or '.'. Values with non-UTF-8 byte sequences must use the BinaryData field. The keys stored in Data must not overlap with the keys in the BinaryData field, this is enforced during validation process.
*
*/
@Export(name="data", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> data;
/**
* @return Data contains the configuration data. Each key must consist of alphanumeric characters, '-', '_' or '.'. Values with non-UTF-8 byte sequences must use the BinaryData field. The keys stored in Data must not overlap with the keys in the BinaryData field, this is enforced during validation process.
*
*/
public Output>> data() {
return Codegen.optional(this.data);
}
/**
* Immutable, if set to true, ensures that data stored in the ConfigMap cannot be updated (only object metadata can be modified). If not set to true, the field can be modified at any time. Defaulted to nil.
*
*/
@Export(name="immutable", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> immutable;
/**
* @return Immutable, if set to true, ensures that data stored in the ConfigMap cannot be updated (only object metadata can be modified). If not set to true, the field can be modified at any time. Defaulted to nil.
*
*/
public Output> immutable() {
return Codegen.optional(this.immutable);
}
/**
* Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kind;
/**
* @return Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*
*/
public Output> kind() {
return Codegen.optional(this.kind);
}
/**
* Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*
*/
@Export(name="metadata", refs={ObjectMetaPatch.class}, tree="[0]")
private Output* @Nullable */ ObjectMetaPatch> metadata;
/**
* @return Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*
*/
public Output> metadata() {
return Codegen.optional(this.metadata);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ConfigMapPatch(String name) {
this(name, ConfigMapPatchArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ConfigMapPatch(String name, @Nullable ConfigMapPatchArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ConfigMapPatch(String name, @Nullable ConfigMapPatchArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("kubernetes:core/v1:ConfigMapPatch", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()));
}
private ConfigMapPatch(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("kubernetes:core/v1:ConfigMapPatch", name, null, makeResourceOptions(options, id));
}
private static ConfigMapPatchArgs makeArgs(@Nullable ConfigMapPatchArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
var builder = args == null ? ConfigMapPatchArgs.builder() : ConfigMapPatchArgs.builder(args);
return builder
.apiVersion("v1")
.kind("ConfigMap")
.build();
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ConfigMapPatch get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ConfigMapPatch(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy