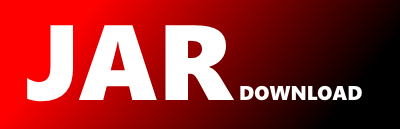
com.pulumi.kubernetes.events.v1.EventPatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.events.v1;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.kubernetes.core.v1.inputs.EventSourcePatchArgs;
import com.pulumi.kubernetes.core.v1.inputs.ObjectReferencePatchArgs;
import com.pulumi.kubernetes.events.v1.inputs.EventSeriesPatchArgs;
import com.pulumi.kubernetes.meta.v1.inputs.ObjectMetaPatchArgs;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EventPatchArgs extends com.pulumi.resources.ResourceArgs {
public static final EventPatchArgs Empty = new EventPatchArgs();
/**
* action is what action was taken/failed regarding to the regarding object. It is machine-readable. This field cannot be empty for new Events and it can have at most 128 characters.
*
*/
@Import(name="action")
private @Nullable Output action;
/**
* @return action is what action was taken/failed regarding to the regarding object. It is machine-readable. This field cannot be empty for new Events and it can have at most 128 characters.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy