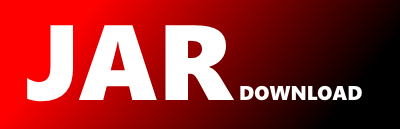
com.pulumi.kubernetes.extensions.v1beta1.outputs.PodSecurityPolicySpec Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.extensions.v1beta1.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.AllowedCSIDriver;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.AllowedFlexVolume;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.AllowedHostPath;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.FSGroupStrategyOptions;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.HostPortRange;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.RunAsGroupStrategyOptions;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.RunAsUserStrategyOptions;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.RuntimeClassStrategyOptions;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.SELinuxStrategyOptions;
import com.pulumi.kubernetes.extensions.v1beta1.outputs.SupplementalGroupsStrategyOptions;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class PodSecurityPolicySpec {
/**
* @return allowPrivilegeEscalation determines if a pod can request to allow privilege escalation. If unspecified, defaults to true.
*
*/
private @Nullable Boolean allowPrivilegeEscalation;
/**
* @return AllowedCSIDrivers is a whitelist of inline CSI drivers that must be explicitly set to be embedded within a pod spec. An empty value indicates that any CSI driver can be used for inline ephemeral volumes.
*
*/
private @Nullable List allowedCSIDrivers;
/**
* @return allowedCapabilities is a list of capabilities that can be requested to add to the container. Capabilities in this field may be added at the pod author's discretion. You must not list a capability in both allowedCapabilities and requiredDropCapabilities.
*
*/
private @Nullable List allowedCapabilities;
/**
* @return allowedFlexVolumes is a whitelist of allowed Flexvolumes. Empty or nil indicates that all Flexvolumes may be used. This parameter is effective only when the usage of the Flexvolumes is allowed in the "volumes" field.
*
*/
private @Nullable List allowedFlexVolumes;
/**
* @return allowedHostPaths is a white list of allowed host paths. Empty indicates that all host paths may be used.
*
*/
private @Nullable List allowedHostPaths;
/**
* @return AllowedProcMountTypes is a whitelist of allowed ProcMountTypes. Empty or nil indicates that only the DefaultProcMountType may be used. This requires the ProcMountType feature flag to be enabled.
*
*/
private @Nullable List allowedProcMountTypes;
/**
* @return allowedUnsafeSysctls is a list of explicitly allowed unsafe sysctls, defaults to none. Each entry is either a plain sysctl name or ends in "*" in which case it is considered as a prefix of allowed sysctls. Single * means all unsafe sysctls are allowed. Kubelet has to whitelist all allowed unsafe sysctls explicitly to avoid rejection.
*
* Examples: e.g. "foo/*" allows "foo/bar", "foo/baz", etc. e.g. "foo.*" allows "foo.bar", "foo.baz", etc.
*
*/
private @Nullable List allowedUnsafeSysctls;
/**
* @return defaultAddCapabilities is the default set of capabilities that will be added to the container unless the pod spec specifically drops the capability. You may not list a capability in both defaultAddCapabilities and requiredDropCapabilities. Capabilities added here are implicitly allowed, and need not be included in the allowedCapabilities list.
*
*/
private @Nullable List defaultAddCapabilities;
/**
* @return defaultAllowPrivilegeEscalation controls the default setting for whether a process can gain more privileges than its parent process.
*
*/
private @Nullable Boolean defaultAllowPrivilegeEscalation;
/**
* @return forbiddenSysctls is a list of explicitly forbidden sysctls, defaults to none. Each entry is either a plain sysctl name or ends in "*" in which case it is considered as a prefix of forbidden sysctls. Single * means all sysctls are forbidden.
*
* Examples: e.g. "foo/*" forbids "foo/bar", "foo/baz", etc. e.g. "foo.*" forbids "foo.bar", "foo.baz", etc.
*
*/
private @Nullable List forbiddenSysctls;
/**
* @return fsGroup is the strategy that will dictate what fs group is used by the SecurityContext.
*
*/
private FSGroupStrategyOptions fsGroup;
/**
* @return hostIPC determines if the policy allows the use of HostIPC in the pod spec.
*
*/
private @Nullable Boolean hostIPC;
/**
* @return hostNetwork determines if the policy allows the use of HostNetwork in the pod spec.
*
*/
private @Nullable Boolean hostNetwork;
/**
* @return hostPID determines if the policy allows the use of HostPID in the pod spec.
*
*/
private @Nullable Boolean hostPID;
/**
* @return hostPorts determines which host port ranges are allowed to be exposed.
*
*/
private @Nullable List hostPorts;
/**
* @return privileged determines if a pod can request to be run as privileged.
*
*/
private @Nullable Boolean privileged;
/**
* @return readOnlyRootFilesystem when set to true will force containers to run with a read only root file system. If the container specifically requests to run with a non-read only root file system the PSP should deny the pod. If set to false the container may run with a read only root file system if it wishes but it will not be forced to.
*
*/
private @Nullable Boolean readOnlyRootFilesystem;
/**
* @return requiredDropCapabilities are the capabilities that will be dropped from the container. These are required to be dropped and cannot be added.
*
*/
private @Nullable List requiredDropCapabilities;
/**
* @return RunAsGroup is the strategy that will dictate the allowable RunAsGroup values that may be set. If this field is omitted, the pod's RunAsGroup can take any value. This field requires the RunAsGroup feature gate to be enabled.
*
*/
private @Nullable RunAsGroupStrategyOptions runAsGroup;
/**
* @return runAsUser is the strategy that will dictate the allowable RunAsUser values that may be set.
*
*/
private RunAsUserStrategyOptions runAsUser;
/**
* @return runtimeClass is the strategy that will dictate the allowable RuntimeClasses for a pod. If this field is omitted, the pod's runtimeClassName field is unrestricted. Enforcement of this field depends on the RuntimeClass feature gate being enabled.
*
*/
private @Nullable RuntimeClassStrategyOptions runtimeClass;
/**
* @return seLinux is the strategy that will dictate the allowable labels that may be set.
*
*/
private SELinuxStrategyOptions seLinux;
/**
* @return supplementalGroups is the strategy that will dictate what supplemental groups are used by the SecurityContext.
*
*/
private SupplementalGroupsStrategyOptions supplementalGroups;
/**
* @return volumes is a white list of allowed volume plugins. Empty indicates that no volumes may be used. To allow all volumes you may use '*'.
*
*/
private @Nullable List volumes;
private PodSecurityPolicySpec() {}
/**
* @return allowPrivilegeEscalation determines if a pod can request to allow privilege escalation. If unspecified, defaults to true.
*
*/
public Optional allowPrivilegeEscalation() {
return Optional.ofNullable(this.allowPrivilegeEscalation);
}
/**
* @return AllowedCSIDrivers is a whitelist of inline CSI drivers that must be explicitly set to be embedded within a pod spec. An empty value indicates that any CSI driver can be used for inline ephemeral volumes.
*
*/
public List allowedCSIDrivers() {
return this.allowedCSIDrivers == null ? List.of() : this.allowedCSIDrivers;
}
/**
* @return allowedCapabilities is a list of capabilities that can be requested to add to the container. Capabilities in this field may be added at the pod author's discretion. You must not list a capability in both allowedCapabilities and requiredDropCapabilities.
*
*/
public List allowedCapabilities() {
return this.allowedCapabilities == null ? List.of() : this.allowedCapabilities;
}
/**
* @return allowedFlexVolumes is a whitelist of allowed Flexvolumes. Empty or nil indicates that all Flexvolumes may be used. This parameter is effective only when the usage of the Flexvolumes is allowed in the "volumes" field.
*
*/
public List allowedFlexVolumes() {
return this.allowedFlexVolumes == null ? List.of() : this.allowedFlexVolumes;
}
/**
* @return allowedHostPaths is a white list of allowed host paths. Empty indicates that all host paths may be used.
*
*/
public List allowedHostPaths() {
return this.allowedHostPaths == null ? List.of() : this.allowedHostPaths;
}
/**
* @return AllowedProcMountTypes is a whitelist of allowed ProcMountTypes. Empty or nil indicates that only the DefaultProcMountType may be used. This requires the ProcMountType feature flag to be enabled.
*
*/
public List allowedProcMountTypes() {
return this.allowedProcMountTypes == null ? List.of() : this.allowedProcMountTypes;
}
/**
* @return allowedUnsafeSysctls is a list of explicitly allowed unsafe sysctls, defaults to none. Each entry is either a plain sysctl name or ends in "*" in which case it is considered as a prefix of allowed sysctls. Single * means all unsafe sysctls are allowed. Kubelet has to whitelist all allowed unsafe sysctls explicitly to avoid rejection.
*
* Examples: e.g. "foo/*" allows "foo/bar", "foo/baz", etc. e.g. "foo.*" allows "foo.bar", "foo.baz", etc.
*
*/
public List allowedUnsafeSysctls() {
return this.allowedUnsafeSysctls == null ? List.of() : this.allowedUnsafeSysctls;
}
/**
* @return defaultAddCapabilities is the default set of capabilities that will be added to the container unless the pod spec specifically drops the capability. You may not list a capability in both defaultAddCapabilities and requiredDropCapabilities. Capabilities added here are implicitly allowed, and need not be included in the allowedCapabilities list.
*
*/
public List defaultAddCapabilities() {
return this.defaultAddCapabilities == null ? List.of() : this.defaultAddCapabilities;
}
/**
* @return defaultAllowPrivilegeEscalation controls the default setting for whether a process can gain more privileges than its parent process.
*
*/
public Optional defaultAllowPrivilegeEscalation() {
return Optional.ofNullable(this.defaultAllowPrivilegeEscalation);
}
/**
* @return forbiddenSysctls is a list of explicitly forbidden sysctls, defaults to none. Each entry is either a plain sysctl name or ends in "*" in which case it is considered as a prefix of forbidden sysctls. Single * means all sysctls are forbidden.
*
* Examples: e.g. "foo/*" forbids "foo/bar", "foo/baz", etc. e.g. "foo.*" forbids "foo.bar", "foo.baz", etc.
*
*/
public List forbiddenSysctls() {
return this.forbiddenSysctls == null ? List.of() : this.forbiddenSysctls;
}
/**
* @return fsGroup is the strategy that will dictate what fs group is used by the SecurityContext.
*
*/
public FSGroupStrategyOptions fsGroup() {
return this.fsGroup;
}
/**
* @return hostIPC determines if the policy allows the use of HostIPC in the pod spec.
*
*/
public Optional hostIPC() {
return Optional.ofNullable(this.hostIPC);
}
/**
* @return hostNetwork determines if the policy allows the use of HostNetwork in the pod spec.
*
*/
public Optional hostNetwork() {
return Optional.ofNullable(this.hostNetwork);
}
/**
* @return hostPID determines if the policy allows the use of HostPID in the pod spec.
*
*/
public Optional hostPID() {
return Optional.ofNullable(this.hostPID);
}
/**
* @return hostPorts determines which host port ranges are allowed to be exposed.
*
*/
public List hostPorts() {
return this.hostPorts == null ? List.of() : this.hostPorts;
}
/**
* @return privileged determines if a pod can request to be run as privileged.
*
*/
public Optional privileged() {
return Optional.ofNullable(this.privileged);
}
/**
* @return readOnlyRootFilesystem when set to true will force containers to run with a read only root file system. If the container specifically requests to run with a non-read only root file system the PSP should deny the pod. If set to false the container may run with a read only root file system if it wishes but it will not be forced to.
*
*/
public Optional readOnlyRootFilesystem() {
return Optional.ofNullable(this.readOnlyRootFilesystem);
}
/**
* @return requiredDropCapabilities are the capabilities that will be dropped from the container. These are required to be dropped and cannot be added.
*
*/
public List requiredDropCapabilities() {
return this.requiredDropCapabilities == null ? List.of() : this.requiredDropCapabilities;
}
/**
* @return RunAsGroup is the strategy that will dictate the allowable RunAsGroup values that may be set. If this field is omitted, the pod's RunAsGroup can take any value. This field requires the RunAsGroup feature gate to be enabled.
*
*/
public Optional runAsGroup() {
return Optional.ofNullable(this.runAsGroup);
}
/**
* @return runAsUser is the strategy that will dictate the allowable RunAsUser values that may be set.
*
*/
public RunAsUserStrategyOptions runAsUser() {
return this.runAsUser;
}
/**
* @return runtimeClass is the strategy that will dictate the allowable RuntimeClasses for a pod. If this field is omitted, the pod's runtimeClassName field is unrestricted. Enforcement of this field depends on the RuntimeClass feature gate being enabled.
*
*/
public Optional runtimeClass() {
return Optional.ofNullable(this.runtimeClass);
}
/**
* @return seLinux is the strategy that will dictate the allowable labels that may be set.
*
*/
public SELinuxStrategyOptions seLinux() {
return this.seLinux;
}
/**
* @return supplementalGroups is the strategy that will dictate what supplemental groups are used by the SecurityContext.
*
*/
public SupplementalGroupsStrategyOptions supplementalGroups() {
return this.supplementalGroups;
}
/**
* @return volumes is a white list of allowed volume plugins. Empty indicates that no volumes may be used. To allow all volumes you may use '*'.
*
*/
public List volumes() {
return this.volumes == null ? List.of() : this.volumes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PodSecurityPolicySpec defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowPrivilegeEscalation;
private @Nullable List allowedCSIDrivers;
private @Nullable List allowedCapabilities;
private @Nullable List allowedFlexVolumes;
private @Nullable List allowedHostPaths;
private @Nullable List allowedProcMountTypes;
private @Nullable List allowedUnsafeSysctls;
private @Nullable List defaultAddCapabilities;
private @Nullable Boolean defaultAllowPrivilegeEscalation;
private @Nullable List forbiddenSysctls;
private FSGroupStrategyOptions fsGroup;
private @Nullable Boolean hostIPC;
private @Nullable Boolean hostNetwork;
private @Nullable Boolean hostPID;
private @Nullable List hostPorts;
private @Nullable Boolean privileged;
private @Nullable Boolean readOnlyRootFilesystem;
private @Nullable List requiredDropCapabilities;
private @Nullable RunAsGroupStrategyOptions runAsGroup;
private RunAsUserStrategyOptions runAsUser;
private @Nullable RuntimeClassStrategyOptions runtimeClass;
private SELinuxStrategyOptions seLinux;
private SupplementalGroupsStrategyOptions supplementalGroups;
private @Nullable List volumes;
public Builder() {}
public Builder(PodSecurityPolicySpec defaults) {
Objects.requireNonNull(defaults);
this.allowPrivilegeEscalation = defaults.allowPrivilegeEscalation;
this.allowedCSIDrivers = defaults.allowedCSIDrivers;
this.allowedCapabilities = defaults.allowedCapabilities;
this.allowedFlexVolumes = defaults.allowedFlexVolumes;
this.allowedHostPaths = defaults.allowedHostPaths;
this.allowedProcMountTypes = defaults.allowedProcMountTypes;
this.allowedUnsafeSysctls = defaults.allowedUnsafeSysctls;
this.defaultAddCapabilities = defaults.defaultAddCapabilities;
this.defaultAllowPrivilegeEscalation = defaults.defaultAllowPrivilegeEscalation;
this.forbiddenSysctls = defaults.forbiddenSysctls;
this.fsGroup = defaults.fsGroup;
this.hostIPC = defaults.hostIPC;
this.hostNetwork = defaults.hostNetwork;
this.hostPID = defaults.hostPID;
this.hostPorts = defaults.hostPorts;
this.privileged = defaults.privileged;
this.readOnlyRootFilesystem = defaults.readOnlyRootFilesystem;
this.requiredDropCapabilities = defaults.requiredDropCapabilities;
this.runAsGroup = defaults.runAsGroup;
this.runAsUser = defaults.runAsUser;
this.runtimeClass = defaults.runtimeClass;
this.seLinux = defaults.seLinux;
this.supplementalGroups = defaults.supplementalGroups;
this.volumes = defaults.volumes;
}
@CustomType.Setter
public Builder allowPrivilegeEscalation(@Nullable Boolean allowPrivilegeEscalation) {
this.allowPrivilegeEscalation = allowPrivilegeEscalation;
return this;
}
@CustomType.Setter
public Builder allowedCSIDrivers(@Nullable List allowedCSIDrivers) {
this.allowedCSIDrivers = allowedCSIDrivers;
return this;
}
public Builder allowedCSIDrivers(AllowedCSIDriver... allowedCSIDrivers) {
return allowedCSIDrivers(List.of(allowedCSIDrivers));
}
@CustomType.Setter
public Builder allowedCapabilities(@Nullable List allowedCapabilities) {
this.allowedCapabilities = allowedCapabilities;
return this;
}
public Builder allowedCapabilities(String... allowedCapabilities) {
return allowedCapabilities(List.of(allowedCapabilities));
}
@CustomType.Setter
public Builder allowedFlexVolumes(@Nullable List allowedFlexVolumes) {
this.allowedFlexVolumes = allowedFlexVolumes;
return this;
}
public Builder allowedFlexVolumes(AllowedFlexVolume... allowedFlexVolumes) {
return allowedFlexVolumes(List.of(allowedFlexVolumes));
}
@CustomType.Setter
public Builder allowedHostPaths(@Nullable List allowedHostPaths) {
this.allowedHostPaths = allowedHostPaths;
return this;
}
public Builder allowedHostPaths(AllowedHostPath... allowedHostPaths) {
return allowedHostPaths(List.of(allowedHostPaths));
}
@CustomType.Setter
public Builder allowedProcMountTypes(@Nullable List allowedProcMountTypes) {
this.allowedProcMountTypes = allowedProcMountTypes;
return this;
}
public Builder allowedProcMountTypes(String... allowedProcMountTypes) {
return allowedProcMountTypes(List.of(allowedProcMountTypes));
}
@CustomType.Setter
public Builder allowedUnsafeSysctls(@Nullable List allowedUnsafeSysctls) {
this.allowedUnsafeSysctls = allowedUnsafeSysctls;
return this;
}
public Builder allowedUnsafeSysctls(String... allowedUnsafeSysctls) {
return allowedUnsafeSysctls(List.of(allowedUnsafeSysctls));
}
@CustomType.Setter
public Builder defaultAddCapabilities(@Nullable List defaultAddCapabilities) {
this.defaultAddCapabilities = defaultAddCapabilities;
return this;
}
public Builder defaultAddCapabilities(String... defaultAddCapabilities) {
return defaultAddCapabilities(List.of(defaultAddCapabilities));
}
@CustomType.Setter
public Builder defaultAllowPrivilegeEscalation(@Nullable Boolean defaultAllowPrivilegeEscalation) {
this.defaultAllowPrivilegeEscalation = defaultAllowPrivilegeEscalation;
return this;
}
@CustomType.Setter
public Builder forbiddenSysctls(@Nullable List forbiddenSysctls) {
this.forbiddenSysctls = forbiddenSysctls;
return this;
}
public Builder forbiddenSysctls(String... forbiddenSysctls) {
return forbiddenSysctls(List.of(forbiddenSysctls));
}
@CustomType.Setter
public Builder fsGroup(FSGroupStrategyOptions fsGroup) {
if (fsGroup == null) {
throw new MissingRequiredPropertyException("PodSecurityPolicySpec", "fsGroup");
}
this.fsGroup = fsGroup;
return this;
}
@CustomType.Setter
public Builder hostIPC(@Nullable Boolean hostIPC) {
this.hostIPC = hostIPC;
return this;
}
@CustomType.Setter
public Builder hostNetwork(@Nullable Boolean hostNetwork) {
this.hostNetwork = hostNetwork;
return this;
}
@CustomType.Setter
public Builder hostPID(@Nullable Boolean hostPID) {
this.hostPID = hostPID;
return this;
}
@CustomType.Setter
public Builder hostPorts(@Nullable List hostPorts) {
this.hostPorts = hostPorts;
return this;
}
public Builder hostPorts(HostPortRange... hostPorts) {
return hostPorts(List.of(hostPorts));
}
@CustomType.Setter
public Builder privileged(@Nullable Boolean privileged) {
this.privileged = privileged;
return this;
}
@CustomType.Setter
public Builder readOnlyRootFilesystem(@Nullable Boolean readOnlyRootFilesystem) {
this.readOnlyRootFilesystem = readOnlyRootFilesystem;
return this;
}
@CustomType.Setter
public Builder requiredDropCapabilities(@Nullable List requiredDropCapabilities) {
this.requiredDropCapabilities = requiredDropCapabilities;
return this;
}
public Builder requiredDropCapabilities(String... requiredDropCapabilities) {
return requiredDropCapabilities(List.of(requiredDropCapabilities));
}
@CustomType.Setter
public Builder runAsGroup(@Nullable RunAsGroupStrategyOptions runAsGroup) {
this.runAsGroup = runAsGroup;
return this;
}
@CustomType.Setter
public Builder runAsUser(RunAsUserStrategyOptions runAsUser) {
if (runAsUser == null) {
throw new MissingRequiredPropertyException("PodSecurityPolicySpec", "runAsUser");
}
this.runAsUser = runAsUser;
return this;
}
@CustomType.Setter
public Builder runtimeClass(@Nullable RuntimeClassStrategyOptions runtimeClass) {
this.runtimeClass = runtimeClass;
return this;
}
@CustomType.Setter
public Builder seLinux(SELinuxStrategyOptions seLinux) {
if (seLinux == null) {
throw new MissingRequiredPropertyException("PodSecurityPolicySpec", "seLinux");
}
this.seLinux = seLinux;
return this;
}
@CustomType.Setter
public Builder supplementalGroups(SupplementalGroupsStrategyOptions supplementalGroups) {
if (supplementalGroups == null) {
throw new MissingRequiredPropertyException("PodSecurityPolicySpec", "supplementalGroups");
}
this.supplementalGroups = supplementalGroups;
return this;
}
@CustomType.Setter
public Builder volumes(@Nullable List volumes) {
this.volumes = volumes;
return this;
}
public Builder volumes(String... volumes) {
return volumes(List.of(volumes));
}
public PodSecurityPolicySpec build() {
final var _resultValue = new PodSecurityPolicySpec();
_resultValue.allowPrivilegeEscalation = allowPrivilegeEscalation;
_resultValue.allowedCSIDrivers = allowedCSIDrivers;
_resultValue.allowedCapabilities = allowedCapabilities;
_resultValue.allowedFlexVolumes = allowedFlexVolumes;
_resultValue.allowedHostPaths = allowedHostPaths;
_resultValue.allowedProcMountTypes = allowedProcMountTypes;
_resultValue.allowedUnsafeSysctls = allowedUnsafeSysctls;
_resultValue.defaultAddCapabilities = defaultAddCapabilities;
_resultValue.defaultAllowPrivilegeEscalation = defaultAllowPrivilegeEscalation;
_resultValue.forbiddenSysctls = forbiddenSysctls;
_resultValue.fsGroup = fsGroup;
_resultValue.hostIPC = hostIPC;
_resultValue.hostNetwork = hostNetwork;
_resultValue.hostPID = hostPID;
_resultValue.hostPorts = hostPorts;
_resultValue.privileged = privileged;
_resultValue.readOnlyRootFilesystem = readOnlyRootFilesystem;
_resultValue.requiredDropCapabilities = requiredDropCapabilities;
_resultValue.runAsGroup = runAsGroup;
_resultValue.runAsUser = runAsUser;
_resultValue.runtimeClass = runtimeClass;
_resultValue.seLinux = seLinux;
_resultValue.supplementalGroups = supplementalGroups;
_resultValue.volumes = volumes;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy