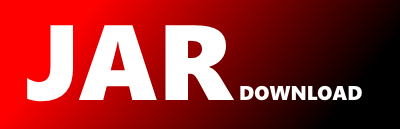
com.pulumi.kubernetes.rbac.v1beta1.inputs.PolicyRulePatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.rbac.v1beta1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* PolicyRule holds information that describes a policy rule, but does not contain information about who the rule applies to or which namespace the rule applies to.
*
*/
public final class PolicyRulePatchArgs extends com.pulumi.resources.ResourceArgs {
public static final PolicyRulePatchArgs Empty = new PolicyRulePatchArgs();
/**
* APIGroups is the name of the APIGroup that contains the resources. If multiple API groups are specified, any action requested against one of the enumerated resources in any API group will be allowed.
*
*/
@Import(name="apiGroups")
private @Nullable Output> apiGroups;
/**
* @return APIGroups is the name of the APIGroup that contains the resources. If multiple API groups are specified, any action requested against one of the enumerated resources in any API group will be allowed.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy