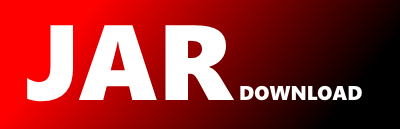
com.pulumi.kubernetes.resource.v1alpha3.ResourceClaim Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.resource.v1alpha3;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import com.pulumi.kubernetes.Utilities;
import com.pulumi.kubernetes.meta.v1.outputs.ObjectMeta;
import com.pulumi.kubernetes.resource.v1alpha3.ResourceClaimArgs;
import com.pulumi.kubernetes.resource.v1alpha3.outputs.ResourceClaimSpec;
import com.pulumi.kubernetes.resource.v1alpha3.outputs.ResourceClaimStatus;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ResourceClaim describes a request for access to resources in the cluster, for use by workloads. For example, if a workload needs an accelerator device with specific properties, this is how that request is expressed. The status stanza tracks whether this claim has been satisfied and what specific resources have been allocated.
*
* This is an alpha type and requires enabling the DynamicResourceAllocation feature gate.
*
*/
@ResourceType(type="kubernetes:resource.k8s.io/v1alpha3:ResourceClaim")
public class ResourceClaim extends com.pulumi.resources.CustomResource {
/**
* APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*
*/
@Export(name="apiVersion", refs={String.class}, tree="[0]")
private Output apiVersion;
/**
* @return APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*
*/
public Output apiVersion() {
return this.apiVersion;
}
/**
* Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*
*/
public Output kind() {
return this.kind;
}
/**
* Standard object metadata
*
*/
@Export(name="metadata", refs={ObjectMeta.class}, tree="[0]")
private Output metadata;
/**
* @return Standard object metadata
*
*/
public Output metadata() {
return this.metadata;
}
/**
* Spec describes what is being requested and how to configure it. The spec is immutable.
*
*/
@Export(name="spec", refs={ResourceClaimSpec.class}, tree="[0]")
private Output spec;
/**
* @return Spec describes what is being requested and how to configure it. The spec is immutable.
*
*/
public Output spec() {
return this.spec;
}
/**
* Status describes whether the claim is ready to use and what has been allocated.
*
*/
@Export(name="status", refs={ResourceClaimStatus.class}, tree="[0]")
private Output* @Nullable */ ResourceClaimStatus> status;
/**
* @return Status describes whether the claim is ready to use and what has been allocated.
*
*/
public Output> status() {
return Codegen.optional(this.status);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ResourceClaim(String name) {
this(name, ResourceClaimArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ResourceClaim(String name, ResourceClaimArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ResourceClaim(String name, ResourceClaimArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("kubernetes:resource.k8s.io/v1alpha3:ResourceClaim", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()));
}
private ResourceClaim(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("kubernetes:resource.k8s.io/v1alpha3:ResourceClaim", name, null, makeResourceOptions(options, id));
}
private static ResourceClaimArgs makeArgs(ResourceClaimArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
var builder = args == null ? ResourceClaimArgs.builder() : ResourceClaimArgs.builder(args);
return builder
.apiVersion("resource.k8s.io/v1alpha3")
.kind("ResourceClaim")
.build();
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("kubernetes:resource.k8s.io/v1alpha1:ResourceClaim").build()),
Output.of(Alias.builder().type("kubernetes:resource.k8s.io/v1alpha2:ResourceClaim").build()),
Output.of(Alias.builder().type("kubernetes:resource.k8s.io/v1beta1:ResourceClaim").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ResourceClaim get(String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ResourceClaim(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy