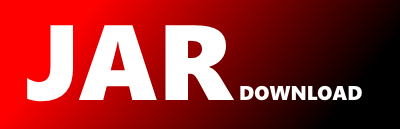
com.pulumi.kubernetes.settings.v1alpha1.inputs.PodPresetSpecPatchArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of kubernetes Show documentation
Show all versions of kubernetes Show documentation
A Pulumi package for creating and managing Kubernetes resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.kubernetes.settings.v1alpha1.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.kubernetes.core.v1.inputs.EnvFromSourcePatchArgs;
import com.pulumi.kubernetes.core.v1.inputs.EnvVarPatchArgs;
import com.pulumi.kubernetes.core.v1.inputs.VolumeMountPatchArgs;
import com.pulumi.kubernetes.core.v1.inputs.VolumePatchArgs;
import com.pulumi.kubernetes.meta.v1.inputs.LabelSelectorPatchArgs;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* PodPresetSpec is a description of a pod preset.
*
*/
public final class PodPresetSpecPatchArgs extends com.pulumi.resources.ResourceArgs {
public static final PodPresetSpecPatchArgs Empty = new PodPresetSpecPatchArgs();
/**
* Env defines the collection of EnvVar to inject into containers.
*
*/
@Import(name="env")
private @Nullable Output> env;
/**
* @return Env defines the collection of EnvVar to inject into containers.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy